Statistical simulation of Sea and Swell parameters
Contents
9. Statistical simulation of Sea and Swell parameters#
Simulate wave parameters for each DWT
inputs required:
historical AWTs
historical sea parameters for each DWT
historical swell (snakes) parameters for each DWT
in this notebook:
Synthetic simulation of sea parameters using Gaussian copulas for each DWT and AWT
Synthetic simulation of snake parameters using k-nearest neighbors algorithm for each DWT and directional sector
Workflow:
Simulating new combinations of wave parameters is accomplished by using copula simulations (Rueda et al. 2016) in the case of seas and the k-nearest neighbors algorithm with random probabilities in the case of swells.
Seas: If there are less than 5 data for a specific DWT/AWT combination, historical values are repeated. If there are less than 50 data for a specific DWT/AWT combination, copula simulation is made with empirical kernel. If there are more than 50 data for a specific DWT/AWT combination, copula simulation is made with kernel density. estimation.
Swells: For each directional sector, 10-nearest neighbors are identified and assigned random probabilites. Synthetic values are obtained from this weighted mean of near points.
import warnings
warnings.filterwarnings('ignore')
import xarray as xr
import numpy as np
import os
import os.path as op
import sys
import pickle as pk
import random
import matplotlib.pyplot as plt
from tabulate import tabulate
sys.path.insert(0, op.join(os.path.abspath(''), '..', '..', '..', '..'))
# teslakit
from bluemath.teslakit2.plotting.waves import Plot_Params_HISTvsSIM_histogram, Plot_Params_HISTvsSIM
from bluemath.teslakit2.waves.waves_params_sim import sea_copula_sim, snakes_k_nearest_sim
Warning: ecCodes 2.21.0 or higher is recommended. You are running version 2.20.0
9.1. Files and paths#
# project path
p_data = r'/media/administrador/HD2/SamoaTonga/data'
site = 'Samoa'
p_site = op.join(p_data, site)
# deliverable folder
p_deliv = op.join(p_site, 'd09_TESLA')
# output path
p_out = op.join(p_deliv,'WAVES')
if not os.path.isdir(p_out): os.makedirs(p_out)
# input data
awt_his_file = op.join(p_deliv, 'SST', 'SST_KMA.nc')
swells_params_file = op.join(p_out, 'Snakes_Parameters_dwt.nc')
seas_params_file = op.join(p_out, 'Sea_partition_dwt.nc')
# output data
swell_sim_params_file = op.join(p_out, 'Snakes_Parameters_copula_sim.nc')
sea_sim_params_file = op.join(p_out, 'Sea_Parameters_copula_sim.nc')
9.2. Parameters#
# load data
snakes_params = xr.open_dataset(swells_params_file)
sea_states = xr.open_dataset(seas_params_file)
awt_his = xr.open_dataset(awt_his_file)
# drop not needed variables
snakes_params = snakes_params.drop({'Fp','Dspr','sdp1','Gamma', 'sg1', 'Tm','sd1'})
# number of random generation
num_sim_rnd = 100000
# number of WT
n_clusters = 36
# amplitude of direction bins (degrees)
amp_dir=30
9.3. SEA States. Copula simulation for each DWT conditioned to each AWT#
# add AWT variable
AWT_his = xr.Dataset({'bmus':(('time'), awt_his.bmus.values.astype(int))},
coords = {'time':(('time'), awt_his.time.values)})
AWT_his = AWT_his.resample(time='1D').pad() # AWT to daily scale
AWT_his = AWT_his.sel(time=slice(sea_states.time.values[0], sea_states.time.values[-1]))
sea_states = sea_states.sel(time=slice(AWT_his.time.values[0], AWT_his.time.values[-1]))
sea_states['awt'] = (('time'), AWT_his.bmus.values)
# initialize output variables
n_data = np.zeros((n_clusters, 6))*np.nan
sim_params = xr.Dataset(
{
'hs':(('awt','bmus','sim'), np.zeros((6, n_clusters, num_sim_rnd))*np.nan),
'tp':(('awt','bmus','sim'), np.zeros((6, n_clusters, num_sim_rnd))*np.nan),
'dpm':(('awt','bmus','sim'), np.zeros((6, n_clusters, num_sim_rnd))*np.nan),
},
coords = {
'sim':(('sim'), np.arange(num_sim_rnd)),
'bmus':(('bmus'), np.arange(n_clusters)),
'awt':(('awt'), np.arange(6))
},
)
# delete nans (hs=0, tp=NaN)
sea_states = sea_states.where(~np.isnan(sea_states.tp),drop=True) # hay 13 días de NaNs(en 20 años)
sea_states = sea_states.where(~np.isnan(sea_states.dpm), drop=True) # el ultimo valor de dir es nan
# keep data from DWT 1-36 (no TCs)
sea_states = sea_states.where(sea_states.bmus<n_clusters, drop=True)
# copula simulation for each DWT and AWT
kernels = ['KDE', 'KDE', 'ECDF'] # empirical dir
n_data, sim_params = sea_copula_sim(n_clusters, sea_states, n_data, sim_params, num_sim_rnd, kernels)
# save
pk.dump(n_data, open(os.path.join(p_out,'n_data_copula_seas.pkl'),"wb"))
sim_params.to_netcdf(sea_sim_params_file)
#n_data = pk.load(open(os.path.join(p_out,'n_data_copula_seas.pkl'),'rb'))
#sim_params= xr.open_dataset(sea_sim_params_file)
# plot number of historical data for each dwt
for aa in range(6):
n_data_awt = n_data[:,aa]
plt.figure(figsize=(10,5))
plt.plot(n_data_awt,'.-')
plt.xlabel('DWT')
plt.xticks(np.arange(0,n_clusters,5),np.arange(1,n_clusters+1,5))
plt.ylabel('nº of sea states (daily)')
plt.grid()
plt.title('AWT ' + str(aa+1))
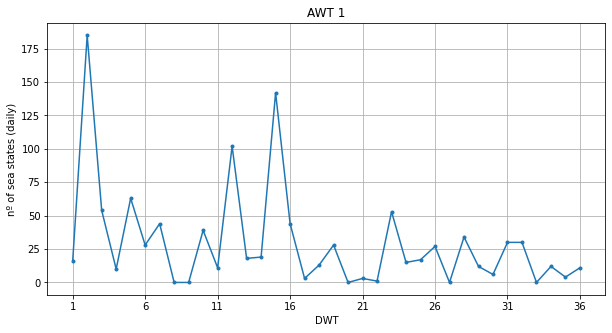
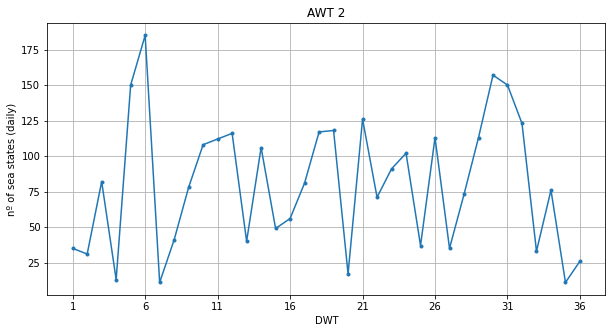
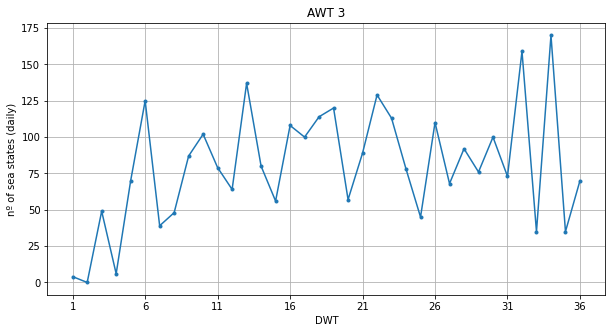
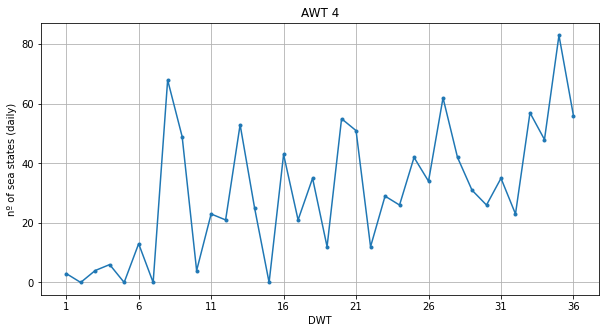
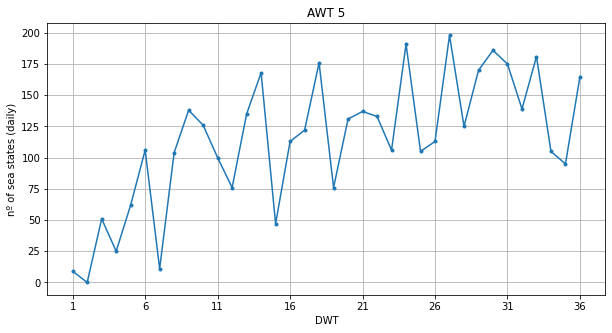
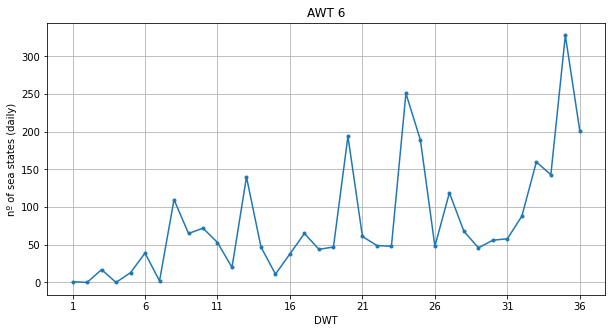
# number of seas for dwt and awt
mydata = np.insert(n_data, 0, np.arange(1,n_clusters+1), axis=1)
# create header
head=['DWT']
for awt in range(1,7):
head.append('AWT ' + str(awt) )
# display table
print('number of historical seas for each AWT and DWT')
print(tabulate(mydata, headers=head, tablefmt="grid"))
number of historical seas for each AWT and DWT
+-------+---------+---------+---------+---------+---------+---------+
| DWT | AWT 1 | AWT 2 | AWT 3 | AWT 4 | AWT 5 | AWT 6 |
+=======+=========+=========+=========+=========+=========+=========+
| 1 | 16 | 35 | 4 | 3 | 9 | 1 |
+-------+---------+---------+---------+---------+---------+---------+
| 2 | 185 | 31 | 0 | 0 | 0 | 0 |
+-------+---------+---------+---------+---------+---------+---------+
| 3 | 54 | 82 | 49 | 4 | 51 | 17 |
+-------+---------+---------+---------+---------+---------+---------+
| 4 | 10 | 13 | 6 | 6 | 25 | 0 |
+-------+---------+---------+---------+---------+---------+---------+
| 5 | 63 | 150 | 70 | 0 | 62 | 13 |
+-------+---------+---------+---------+---------+---------+---------+
| 6 | 28 | 185 | 125 | 13 | 106 | 39 |
+-------+---------+---------+---------+---------+---------+---------+
| 7 | 44 | 11 | 39 | 0 | 11 | 2 |
+-------+---------+---------+---------+---------+---------+---------+
| 8 | 0 | 41 | 48 | 68 | 104 | 110 |
+-------+---------+---------+---------+---------+---------+---------+
| 9 | 0 | 78 | 87 | 49 | 138 | 65 |
+-------+---------+---------+---------+---------+---------+---------+
| 10 | 39 | 108 | 102 | 4 | 126 | 72 |
+-------+---------+---------+---------+---------+---------+---------+
| 11 | 11 | 112 | 79 | 23 | 100 | 53 |
+-------+---------+---------+---------+---------+---------+---------+
| 12 | 102 | 116 | 64 | 21 | 76 | 20 |
+-------+---------+---------+---------+---------+---------+---------+
| 13 | 18 | 40 | 137 | 53 | 135 | 140 |
+-------+---------+---------+---------+---------+---------+---------+
| 14 | 19 | 106 | 80 | 25 | 168 | 47 |
+-------+---------+---------+---------+---------+---------+---------+
| 15 | 142 | 49 | 56 | 0 | 47 | 11 |
+-------+---------+---------+---------+---------+---------+---------+
| 16 | 44 | 56 | 108 | 43 | 113 | 38 |
+-------+---------+---------+---------+---------+---------+---------+
| 17 | 3 | 81 | 100 | 21 | 122 | 65 |
+-------+---------+---------+---------+---------+---------+---------+
| 18 | 13 | 117 | 114 | 35 | 176 | 44 |
+-------+---------+---------+---------+---------+---------+---------+
| 19 | 28 | 118 | 120 | 12 | 76 | 47 |
+-------+---------+---------+---------+---------+---------+---------+
| 20 | 0 | 17 | 57 | 55 | 131 | 194 |
+-------+---------+---------+---------+---------+---------+---------+
| 21 | 3 | 126 | 89 | 51 | 137 | 61 |
+-------+---------+---------+---------+---------+---------+---------+
| 22 | 1 | 71 | 129 | 12 | 133 | 49 |
+-------+---------+---------+---------+---------+---------+---------+
| 23 | 53 | 91 | 113 | 29 | 106 | 48 |
+-------+---------+---------+---------+---------+---------+---------+
| 24 | 15 | 102 | 78 | 26 | 191 | 251 |
+-------+---------+---------+---------+---------+---------+---------+
| 25 | 17 | 37 | 45 | 42 | 105 | 189 |
+-------+---------+---------+---------+---------+---------+---------+
| 26 | 27 | 113 | 110 | 34 | 113 | 48 |
+-------+---------+---------+---------+---------+---------+---------+
| 27 | 0 | 35 | 68 | 62 | 198 | 119 |
+-------+---------+---------+---------+---------+---------+---------+
| 28 | 34 | 73 | 92 | 42 | 125 | 68 |
+-------+---------+---------+---------+---------+---------+---------+
| 29 | 12 | 113 | 76 | 31 | 170 | 46 |
+-------+---------+---------+---------+---------+---------+---------+
| 30 | 6 | 157 | 100 | 26 | 186 | 56 |
+-------+---------+---------+---------+---------+---------+---------+
| 31 | 30 | 150 | 73 | 35 | 175 | 58 |
+-------+---------+---------+---------+---------+---------+---------+
| 32 | 30 | 123 | 159 | 23 | 139 | 88 |
+-------+---------+---------+---------+---------+---------+---------+
| 33 | 0 | 33 | 35 | 57 | 181 | 160 |
+-------+---------+---------+---------+---------+---------+---------+
| 34 | 12 | 76 | 170 | 48 | 105 | 143 |
+-------+---------+---------+---------+---------+---------+---------+
| 35 | 4 | 11 | 35 | 83 | 95 | 328 |
+-------+---------+---------+---------+---------+---------+---------+
| 36 | 11 | 26 | 70 | 56 | 165 | 201 |
+-------+---------+---------+---------+---------+---------+---------+
# plot all data (all DWTs)
# variables to plot
d_lab = {
'hs': 'Hs (m)',
'tp': 'Tp (s)',
'dpm': 'Dir (º)',
}
# Historical vs Simulated: histogram parameters
Plot_Params_HISTvsSIM_histogram(sea_states, sim_params, d_lab);
# Historical vs Simulated: scatter plot parameters
Plot_Params_HISTvsSIM(sea_states, sim_params, d_lab);
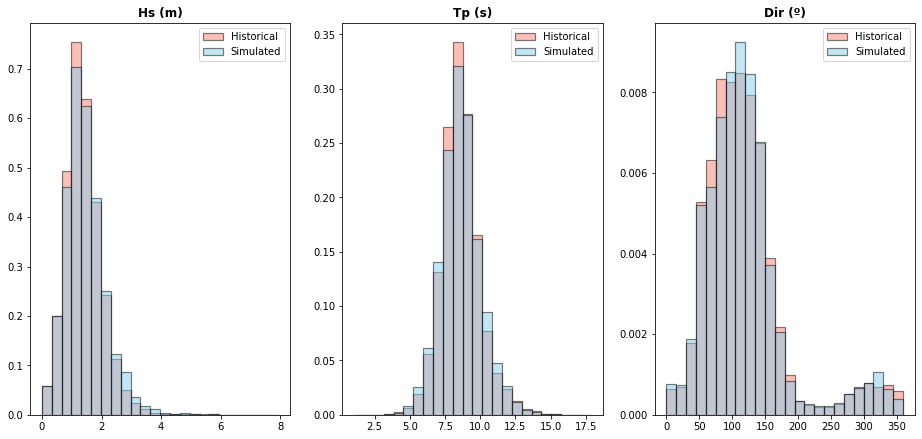
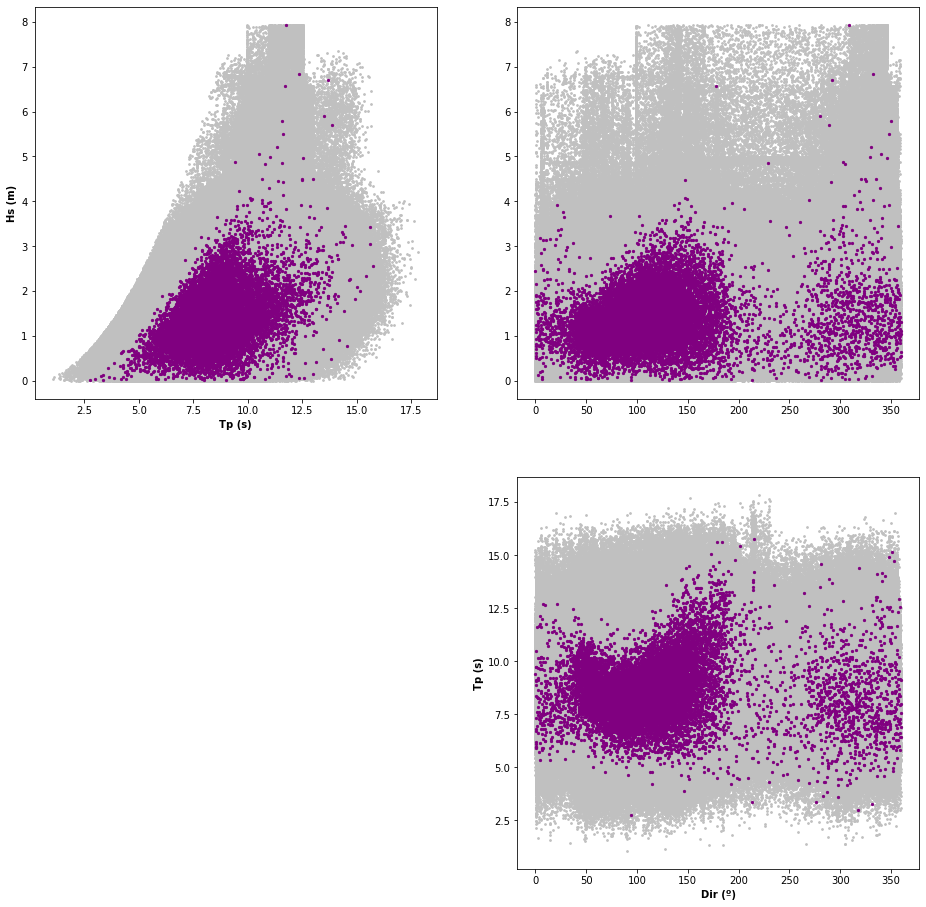
# select DWT to plot for all AWTs
dwt=10
sea_states_d = sea_states.where(sea_states.bmus==dwt, drop=True)
for aa in range(6):
sea_states_d_aa = sea_states_d.where(sea_states.bmus==dwt, drop=True)
sea_states_d_aa = sea_states_d_aa.where(sea_states_d_aa.awt==aa, drop=True)
sim_params_d_aa = sim_params.sel(bmus=dwt, awt=aa)
print('AWT ' + str(aa+1) + '. DWT ' + str(dwt+1) + '. ' + str(len(sea_states_d_aa.time)) + ' data')
# variables to plot
d_lab = {
'hs': 'Hs (m)',
'tp': 'Tp (s)',
'dpm': 'Dir (º)',
}
# Historical vs Simulated: histogram parameters
Plot_Params_HISTvsSIM_histogram(sea_states_d_aa, sim_params_d_aa, d_lab);
# Historical vs Simulated: scatter plot parameters
Plot_Params_HISTvsSIM(sea_states_d_aa, sim_params_d_aa, d_lab);
AWT 1. DWT 11. 11 data
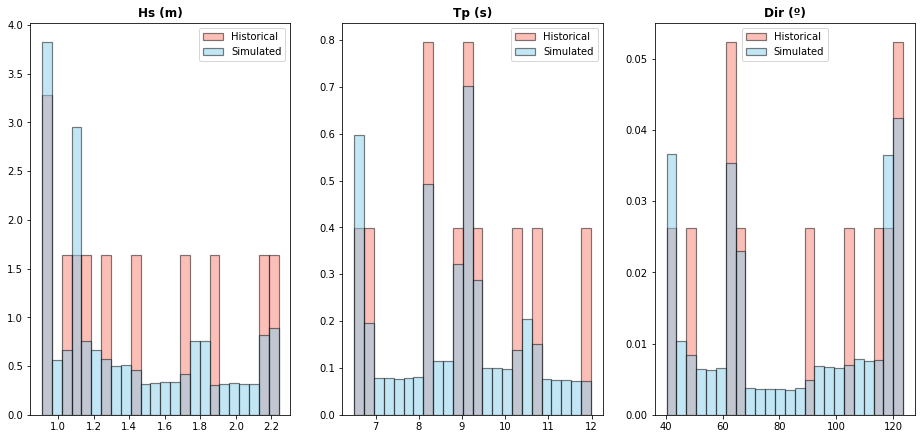
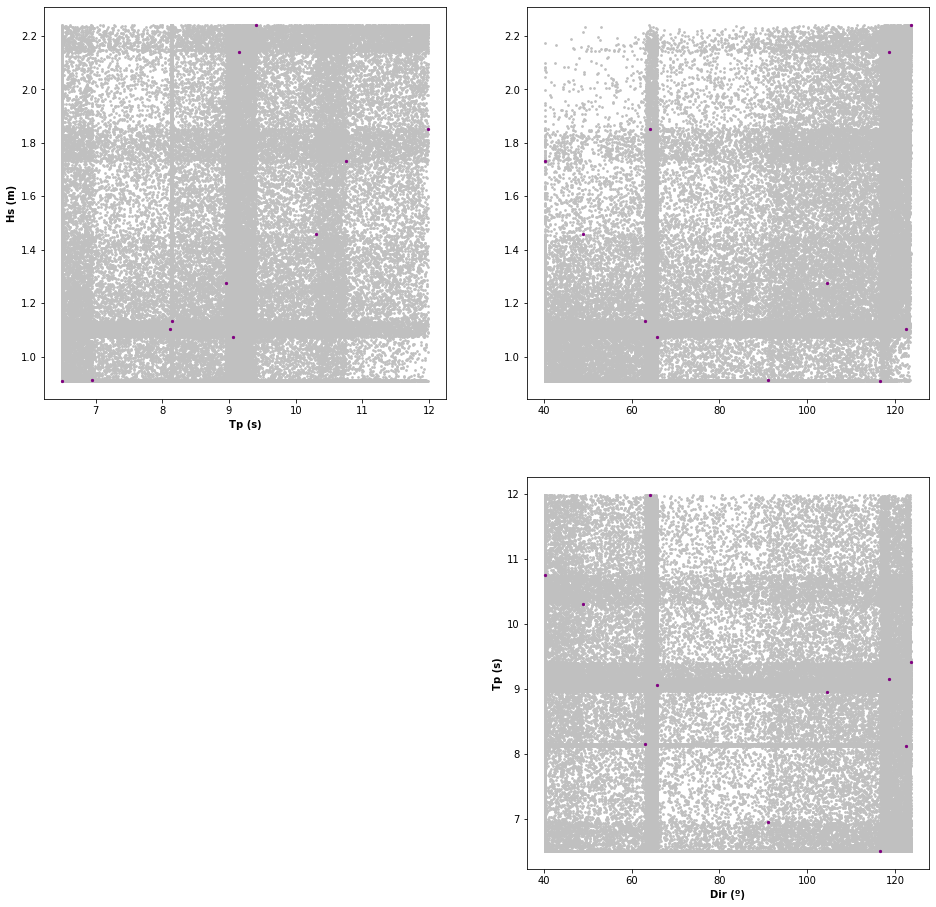
AWT 2. DWT 11. 112 data
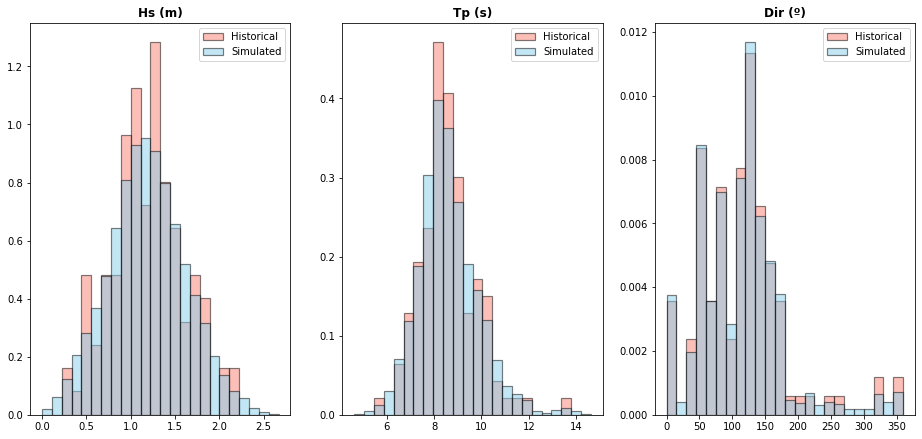
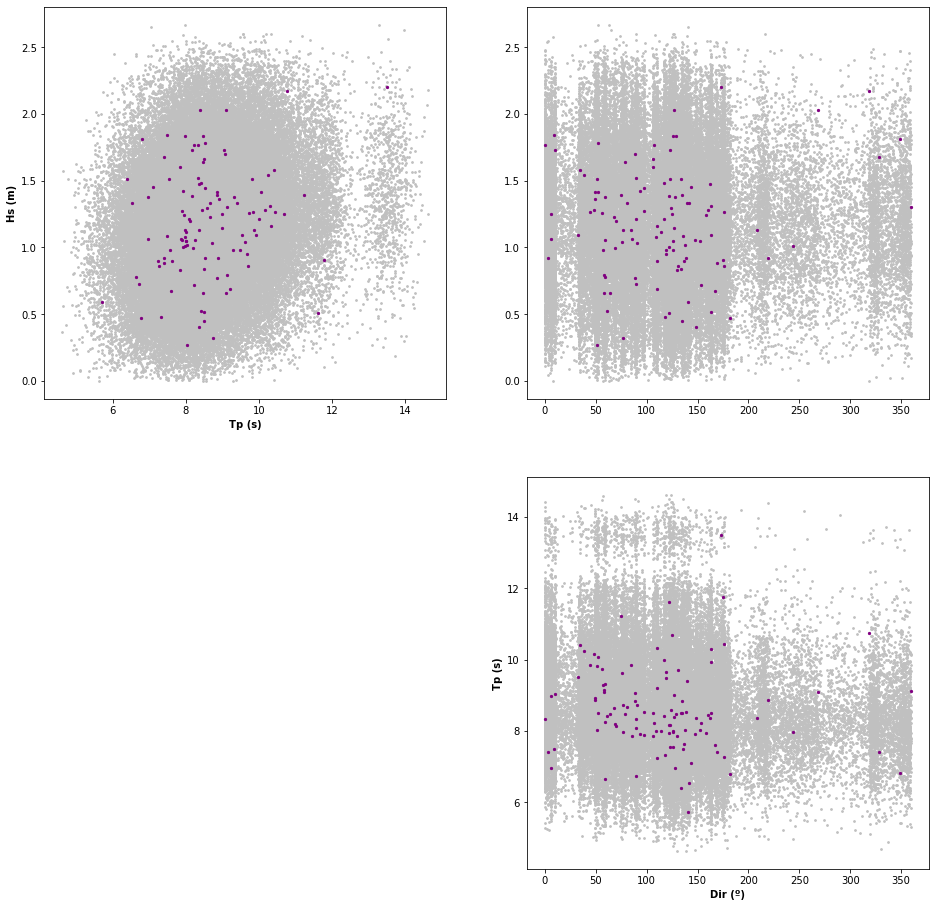
AWT 3. DWT 11. 79 data
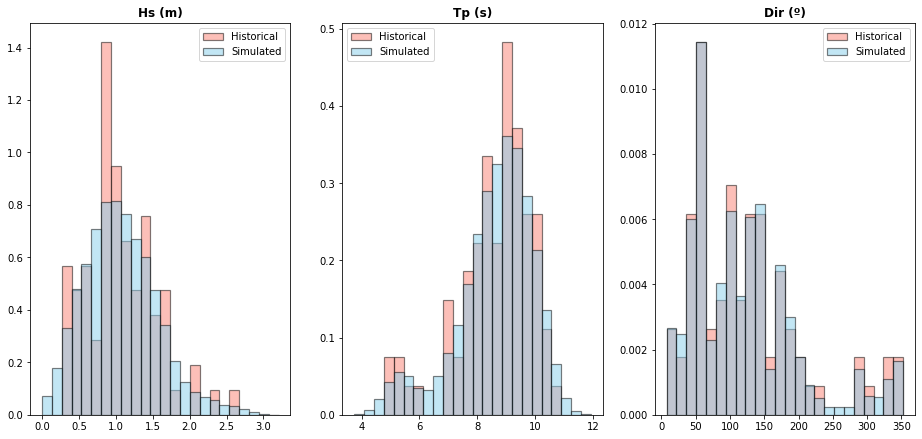
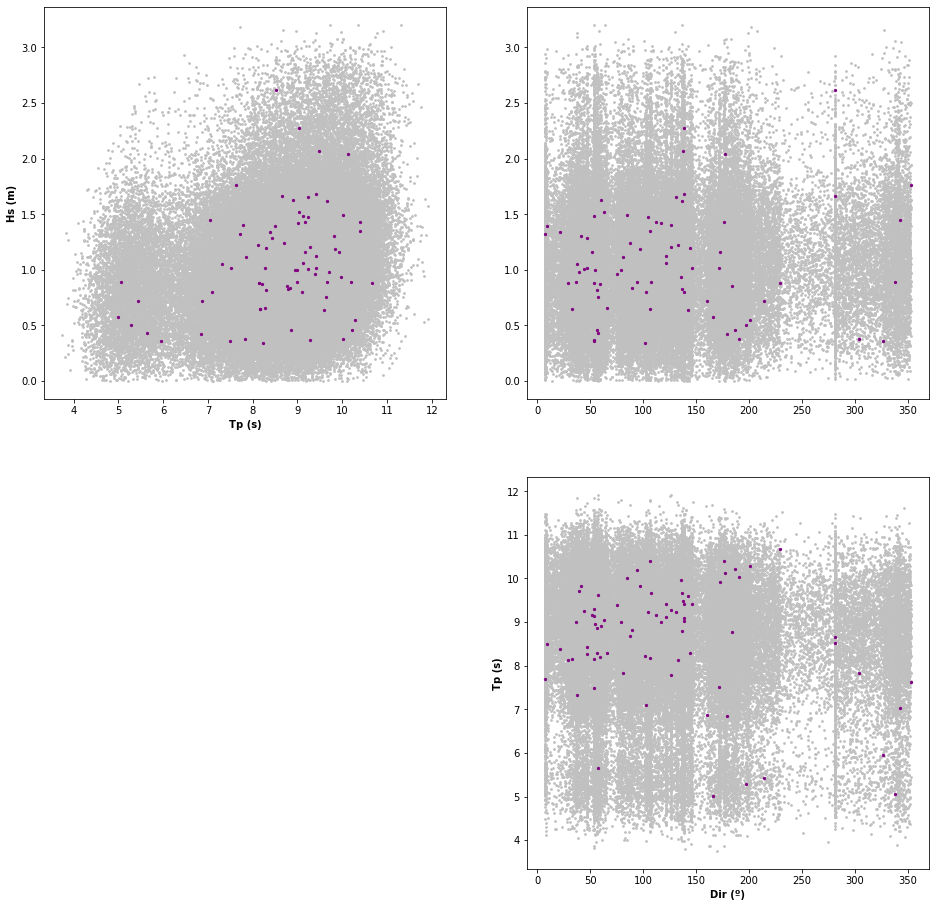
AWT 4. DWT 11. 23 data
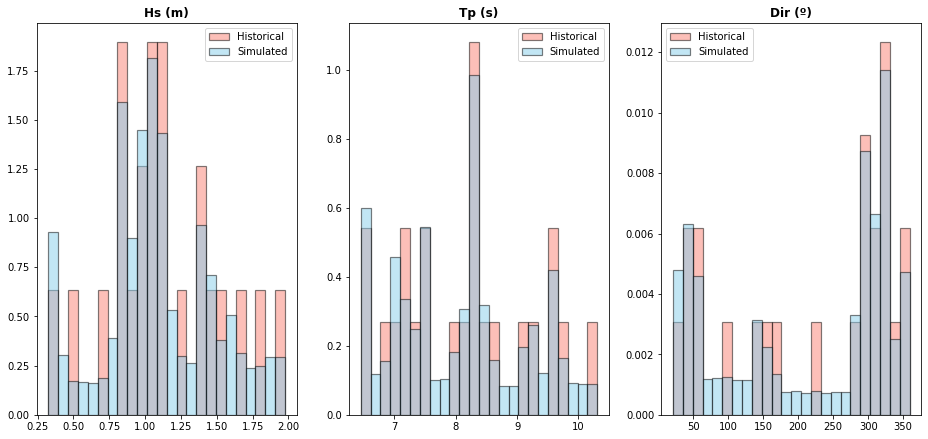
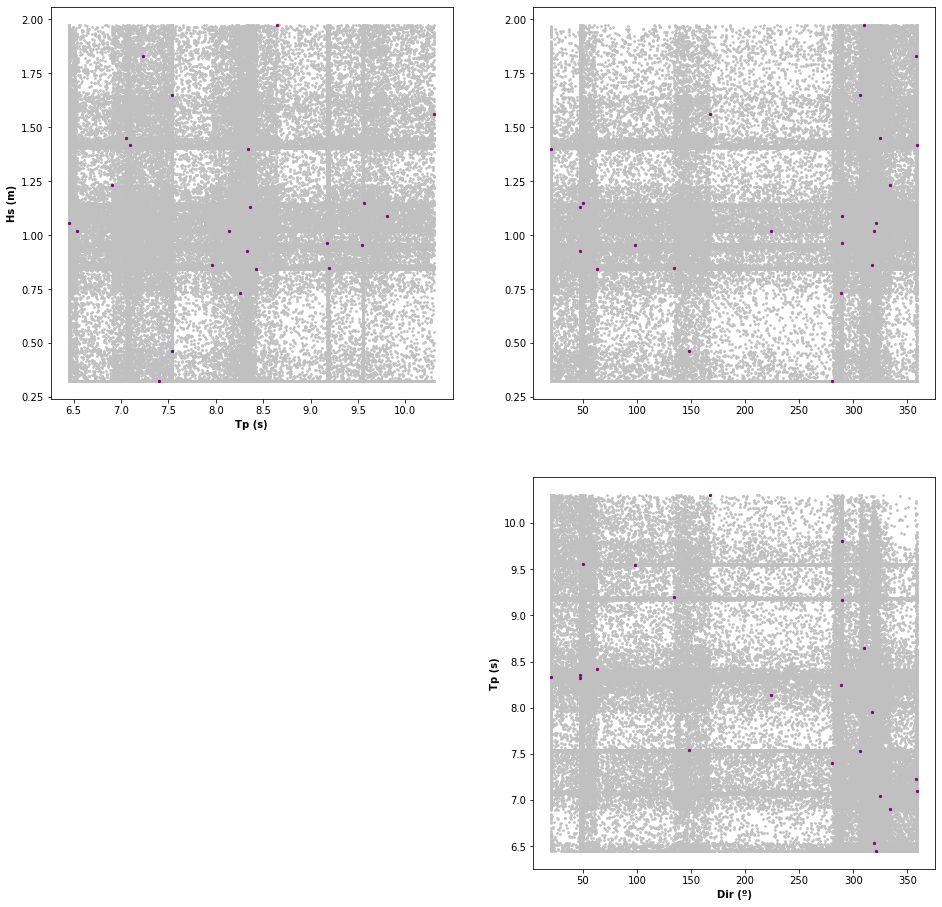
AWT 5. DWT 11. 100 data
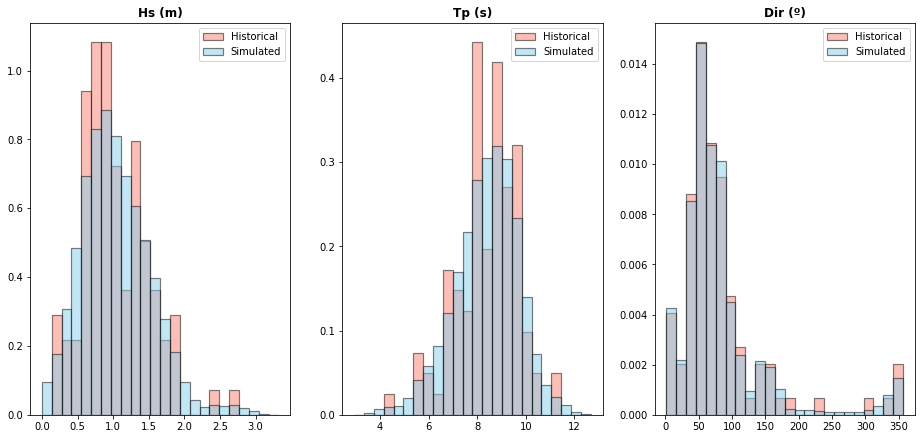
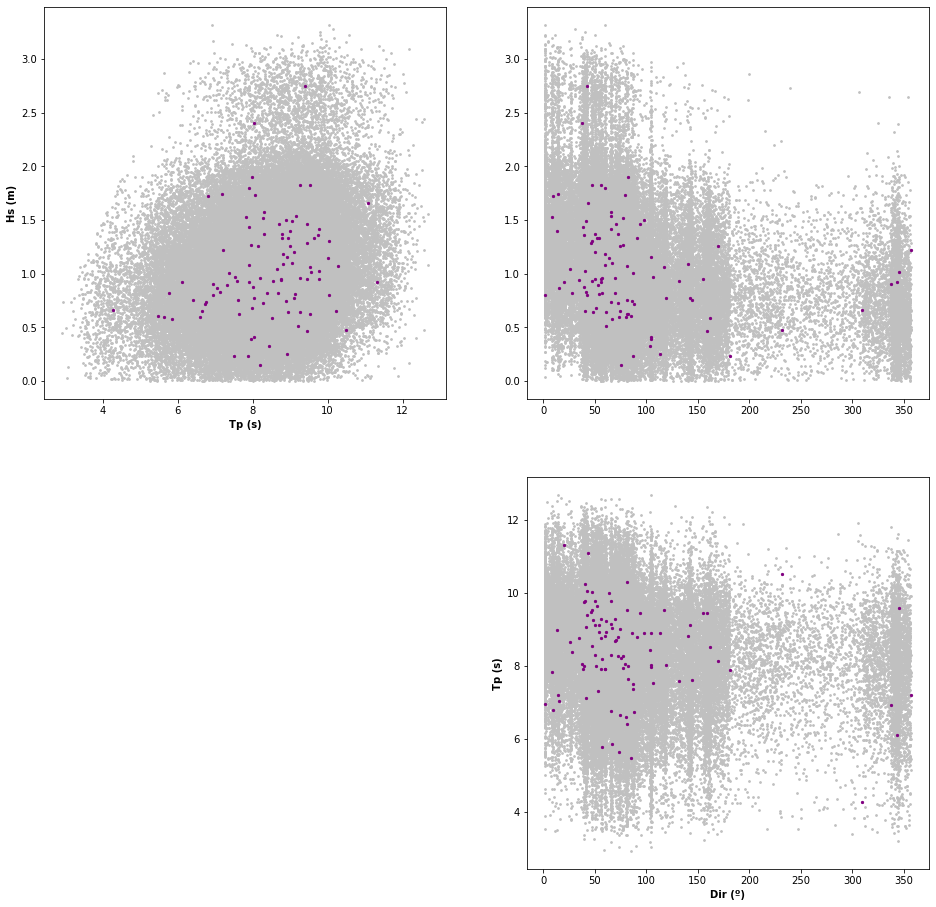
AWT 6. DWT 11. 53 data
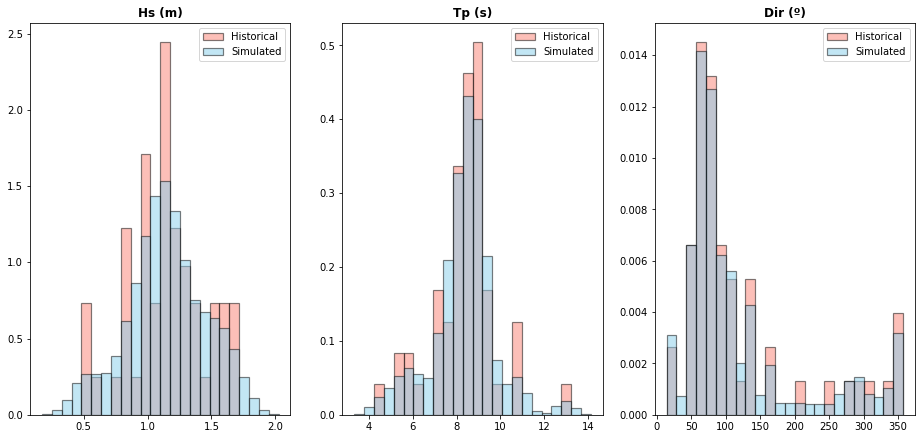
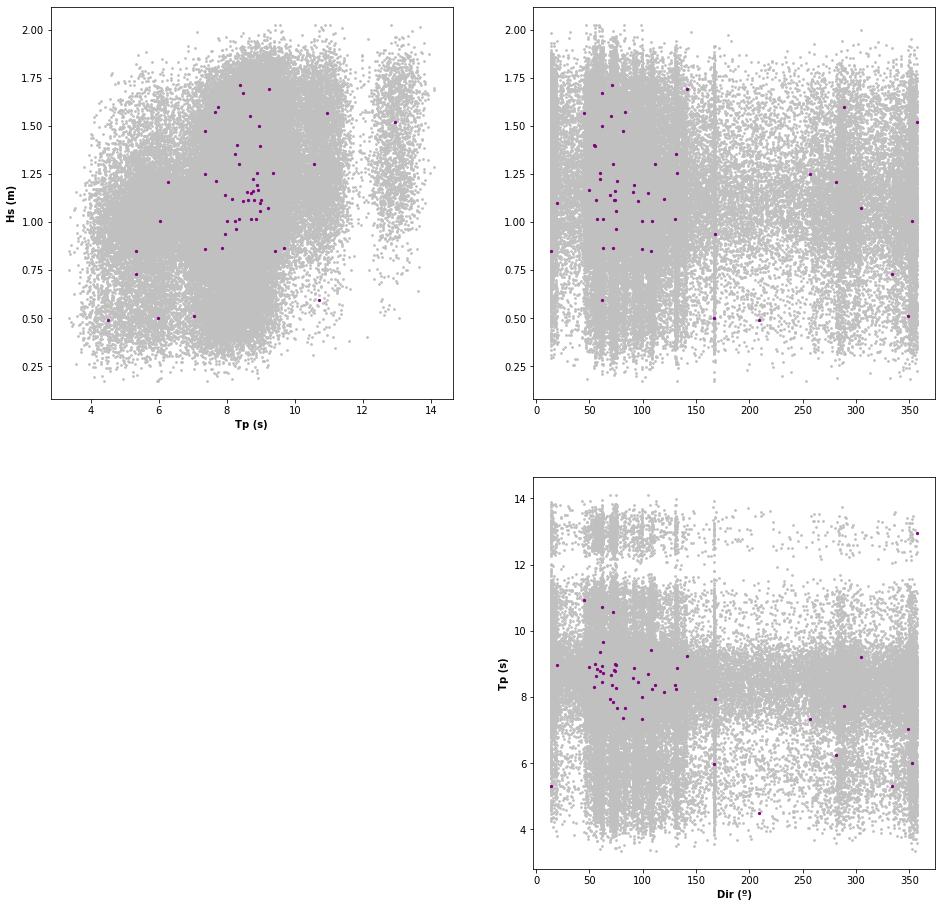
9.4. SWELLS (Snakes). k-nearest simulation for each DWT and for each directional sector#
# bins dir
dir_ini = np.arange(0,360,amp_dir)
dir_end = np.arange(amp_dir,360+amp_dir,amp_dir)
# number of nearest points to each point
k=10
# initialize output variables
n_data = np.zeros((n_clusters, len(dir_ini)))*np.nan
sim_params = xr.Dataset(
{
'Hs':(('wt','dire','sim'), np.zeros((n_clusters, len(dir_ini), num_sim_rnd))*np.nan),
'Tp':(('wt','dire','sim'), np.zeros((n_clusters, len(dir_ini), num_sim_rnd))*np.nan),
'Dir':(('wt','dire','sim'), np.zeros((n_clusters, len(dir_ini), num_sim_rnd))*np.nan),
'Duration':(('wt','dire','sim'), np.zeros((n_clusters, len(dir_ini), num_sim_rnd))*np.nan),
'Tau':(('wt','dire','sim'), np.zeros((n_clusters, len(dir_ini), num_sim_rnd))*np.nan),
'sh1':(('wt','dire','sim'), np.zeros((n_clusters, len(dir_ini), num_sim_rnd))*np.nan),
'sf1':(('wt','dire','sim'), np.zeros((n_clusters, len(dir_ini), num_sim_rnd))*np.nan),
'H_ini':(('wt','dire','sim'), np.zeros((n_clusters, len(dir_ini), num_sim_rnd))*np.nan),
},
coords = {
'sim':(('sim'), np.arange(num_sim_rnd)),
'wt':(('wt'), np.arange(n_clusters)),
'dir_ini':(('dire'), dir_ini),
'dir_end':(('dire'), dir_end),
},
)
# Keep snakes where Hs is greater than H_ini
snakes_params = snakes_params.where(snakes_params.H_ini<=snakes_params.Hs,drop=True)
# Simulate using nearest data from within the directional sector (all DWTs considered)
#n_data, sim_params = snakes_k_nearest_sim(snakes_params, n_data, sim_params, k, dir_ini, dir_end, n_clusters, num_sim_rnd)
# save
#pk.dump(n_data, open(os.path.join(p_out,'n_data_copula_snakes.pkl'),"wb"))
#sim_params.to_netcdf(swell_sim_params_file)
sim_params = xr.open_dataset(swell_sim_params_file)
n_data = pk.load(open(os.path.join(p_out,'n_data_copula_snakes.pkl'),'rb'))
# plot number of historical snakes for each direction and DWT
y_dir=np.append(dir_ini, 360)
x_dwt=np.arange(n_clusters)
plt.figure(figsize=(10,5))
plt.pcolormesh(x_dwt+1, y_dir, n_data.T, cmap='magma_r')
plt.ylabel('directions')
plt.xlabel('DWT')
plt.title('number of historical swells')
plt.colorbar()
<matplotlib.colorbar.Colorbar at 0x12c101f10>
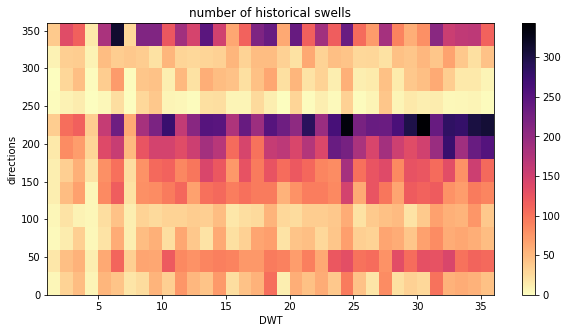
# number of snakes for dwt and direction
mydata = np.insert(n_data, 0, np.arange(1,n_clusters+1), axis=1)
# create header
head=['DWT']
for dir1, dir2 in zip(dir_ini, dir_end):
head.append('dir\n' + str(dir1) + '\n' + str(dir2))
# display table
print('number of historical snakes for each direction and DWT')
print(tabulate(mydata, headers=head, tablefmt="grid"))
number of historical snakes for each direction and DWT
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| DWT | dir | dir | dir | dir | dir | dir | dir | dir | dir | dir | dir | dir |
| | 0 | 30 | 60 | 90 | 120 | 150 | 180 | 210 | 240 | 270 | 300 | 330 |
| | 30 | 60 | 90 | 120 | 150 | 180 | 210 | 240 | 270 | 300 | 330 | 360 |
+=======+=======+=======+=======+=======+=======+=======+=======+=======+=======+=======+=======+=======+
| 1 | 6 | 17 | 3 | 6 | 11 | 11 | 16 | 35 | 2 | 0 | 9 | 38 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 2 | 32 | 46 | 13 | 20 | 47 | 34 | 82 | 104 | 9 | 29 | 34 | 135 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 3 | 47 | 56 | 34 | 9 | 68 | 57 | 70 | 114 | 13 | 46 | 35 | 115 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 4 | 7 | 11 | 5 | 6 | 6 | 19 | 30 | 36 | 0 | 2 | 9 | 16 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 5 | 53 | 62 | 20 | 23 | 84 | 80 | 138 | 159 | 5 | 36 | 47 | 182 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 6 | 42 | 110 | 59 | 44 | 117 | 103 | 158 | 231 | 23 | 72 | 35 | 313 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 7 | 18 | 34 | 12 | 11 | 21 | 23 | 50 | 61 | 0 | 2 | 39 | 27 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 8 | 25 | 65 | 48 | 32 | 80 | 79 | 125 | 184 | 27 | 41 | 36 | 217 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 9 | 54 | 63 | 57 | 26 | 83 | 108 | 147 | 220 | 39 | 43 | 21 | 216 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 10 | 34 | 120 | 24 | 32 | 95 | 113 | 147 | 275 | 8 | 11 | 53 | 122 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 11 | 76 | 85 | 63 | 32 | 109 | 86 | 134 | 161 | 6 | 50 | 30 | 188 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 12 | 51 | 78 | 39 | 35 | 67 | 99 | 153 | 210 | 2 | 21 | 27 | 146 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 13 | 42 | 88 | 20 | 34 | 103 | 141 | 187 | 253 | 22 | 58 | 30 | 248 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 14 | 73 | 92 | 61 | 47 | 108 | 122 | 170 | 250 | 23 | 47 | 33 | 150 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 15 | 23 | 89 | 22 | 17 | 93 | 74 | 107 | 177 | 7 | 44 | 53 | 63 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 16 | 43 | 74 | 33 | 23 | 103 | 128 | 144 | 238 | 7 | 22 | 32 | 113 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 17 | 55 | 75 | 65 | 26 | 94 | 94 | 101 | 192 | 26 | 45 | 48 | 218 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 18 | 104 | 95 | 69 | 54 | 94 | 127 | 159 | 252 | 11 | 63 | 47 | 236 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 19 | 12 | 91 | 19 | 27 | 56 | 105 | 167 | 231 | 0 | 22 | 33 | 62 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 20 | 58 | 70 | 42 | 25 | 79 | 121 | 141 | 204 | 30 | 53 | 21 | 238 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 21 | 45 | 92 | 46 | 36 | 93 | 109 | 173 | 288 | 2 | 19 | 62 | 114 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 22 | 60 | 62 | 28 | 36 | 93 | 88 | 140 | 195 | 11 | 32 | 32 | 188 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 23 | 40 | 123 | 41 | 39 | 85 | 82 | 236 | 264 | 3 | 11 | 46 | 117 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 24 | 95 | 132 | 69 | 60 | 147 | 186 | 225 | 336 | 33 | 57 | 42 | 237 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 25 | 43 | 101 | 34 | 21 | 61 | 103 | 181 | 223 | 2 | 12 | 28 | 107 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 26 | 18 | 105 | 31 | 38 | 125 | 125 | 143 | 237 | 7 | 14 | 29 | 72 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 27 | 83 | 78 | 65 | 45 | 98 | 136 | 187 | 235 | 41 | 45 | 21 | 186 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 28 | 22 | 133 | 60 | 49 | 65 | 85 | 152 | 262 | 7 | 14 | 45 | 89 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 29 | 33 | 105 | 41 | 20 | 119 | 128 | 135 | 298 | 15 | 39 | 41 | 58 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 30 | 27 | 129 | 68 | 38 | 113 | 124 | 149 | 343 | 12 | 46 | 51 | 80 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 31 | 101 | 128 | 82 | 67 | 119 | 106 | 195 | 238 | 13 | 61 | 41 | 208 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 32 | 56 | 141 | 60 | 57 | 79 | 133 | 275 | 284 | 5 | 35 | 66 | 155 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 33 | 59 | 101 | 64 | 54 | 71 | 79 | 189 | 279 | 6 | 16 | 39 | 163 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 34 | 56 | 112 | 58 | 76 | 94 | 153 | 236 | 302 | 7 | 16 | 22 | 166 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 35 | 45 | 108 | 47 | 40 | 88 | 108 | 254 | 309 | 2 | 7 | 44 | 113 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
| 36 | 87 | 118 | 121 | 77 | 112 | 115 | 197 | 237 | 10 | 46 | 29 | 248 |
+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
# select dwt and direction to compare historical and simulated
wt = 4
dire = 11
#for dire in range(12):
dir_ini = np.arange(0,360,amp_dir)
dir_end = np.arange(amp_dir,360+amp_dir,amp_dir)
snakes_params_wt = snakes_params.where(snakes_params.bmus==wt, drop=True)
snakes_params_dir = snakes_params_wt.where((snakes_params_wt.Dir>=dir_ini[dire]) & (snakes_params_wt.Dir<dir_end[dire]), drop=True)
sim_params_dir = sim_params.isel(wt=wt, dire=dire)
print('DWT ' + str(wt+1) + '. Dir ' + str(dir_ini[dire]) + '-' + str(dir_end[dire]) + '. ' + str(len(snakes_params_dir.time)) + ' historical data')
# variables to plot
d_lab = {
'Hs': 'Hs (m)',
'Tp': 'Tp (s)',
'Dir': 'Dir (º)',
}
# Historical vs Simulated: histogram parameters
Plot_Params_HISTvsSIM_histogram(snakes_params_dir, sim_params_dir, d_lab);
# variables to plot
d_lab = {
'Duration': 'duration (h)',
'Tau': 'time until Hs* (h)',
'sh1': 'linear fit Hs*',
'sf1': 'linear fit Tp*',
'H_ini': 'Hs at ini (m)',
}
# Historical vs Simulated: histogram parameters
Plot_Params_HISTvsSIM_histogram(snakes_params_dir, sim_params_dir, d_lab);
# variables to plot
d_lab = {
'Hs': 'Hs (m)',
'Tp': 'Tp (s)',
'Dir': 'Dir (º)',
'Duration': 'duration (h)',
'Tau': 'time until Hs* (h)',
'sh1': 'linear fit Hs*',
'sf1': 'linear fit Tp*',
'H_ini': 'Hs at ini (m)',
}
# Historical vs Simulated: scatter plot parameters
Plot_Params_HISTvsSIM(snakes_params_dir, sim_params_dir, d_lab);
DWT 5. Dir 330-360. 182 historical data
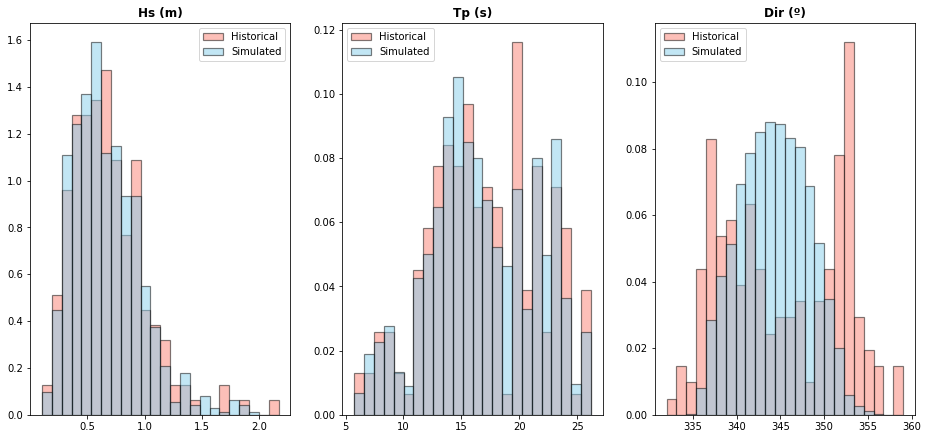
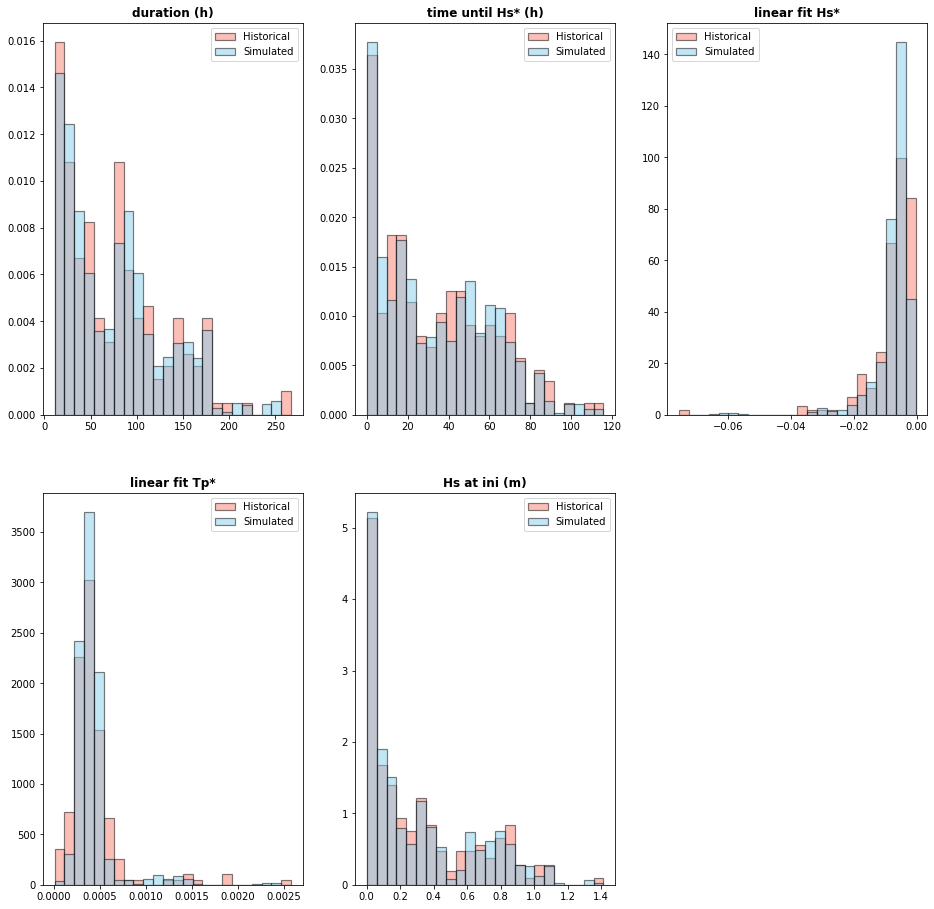
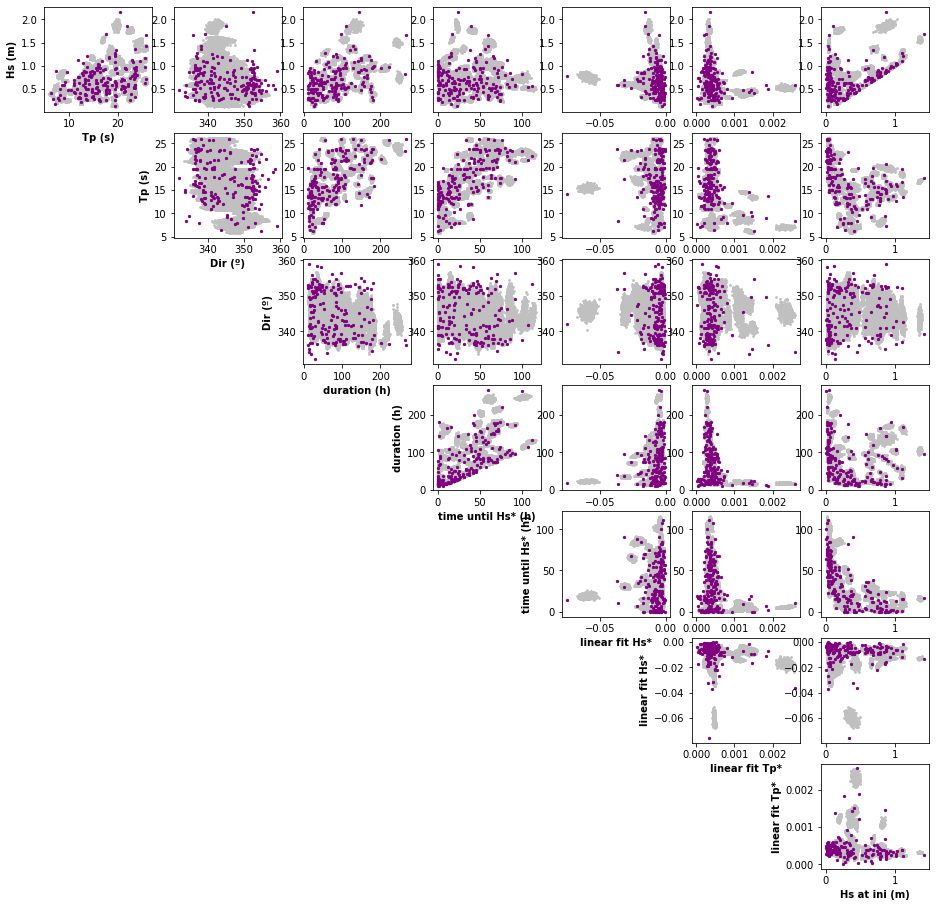