Forecast based on El Niño3.4 forecast and the MJO
Contents
Forecast based on El Niño3.4 forecast and the MJO#
As an alternative and/or complementary method for forecasting the wave spectra, we propose a model based on forecast data of El Niño 3.4 index and the MJO
# common
import warnings
warnings.filterwarnings('ignore')
import os
import os.path as op
import sys
import requests
from IPython.display import Image
# pip
import numpy as np
import xarray as xr
# DEV: bluemath
sys.path.insert(0, op.join(op.abspath(''), '..', '..', '..', '..', '..'))
# bluemath modules
from bluemath.binwaves.nino34 import n34_format, n34_classification
from bluemath.binwaves.plotting.nino34 import Plot_n34_forecast
from bluemath.plotting.spectra import Plot_spec_n34_mjo_month
Database and site parameters#
# database
p_data = r'/media/administrador/HD2/SamoaTonga/data'
site = 'Samoa'
p_site = op.join(p_data, site)
# Madden Julian Oscillation
p_mjo = op.join(p_data, 'mjo.nc')
# nino34 dataset
p_n34 = op.join(p_data, 'ninho34.txt')
# MJO forecast data
p_n34_forecast = op.join(p_data, 'nino34Mon.nc')
# deliverable folder
p_deliv = op.join(p_site, 'd04_seasonal_forecast_swells')
# kma folder
p_kma = op.join(p_deliv, 'kma')
# spectra kma
p_kma_spec_classification = op.join(p_kma, 'spec_KMA_classification.nc') # classified superpoint
Hindcast data
# Load suerpoint with KMeans classification data
sp_mod_daily = xr.open_dataset(p_kma_spec_classification)
# Load mjo
mjo = xr.open_dataset(p_mjo)
# load ninho34 data
n34 = np.loadtxt(p_n34, skiprows=1, max_rows=74)
n34 = n34_format(n34, rolling_mean=False)
# add el nino and la nina events
n34, _, _ = n34_classification(n34, l1=0.5, l2=-0.5)
# resample dataset to daily
n34 = n34.resample(time = '1D').interpolate("linear")
# find common dates for mjo, ninho34 and superpoint datasets
min_d = np.nanmax([mjo.time.values[0], n34.time.values[0], sp_mod_daily.time.values[0]])
max_d = np.nanmin([mjo.time.values[-1], n34.time.values[-1], sp_mod_daily.time.values[-1]])
n34 = n34.sel(time = slice(min_d, max_d))
mjo = mjo.sel(time = slice(min_d, max_d))
sp_mod_daily = sp_mod_daily.sel(time = slice(min_d, max_d))
# add mjo and ninho34 variables to superpoint classification
sp_mod_daily['mjo'] = ('time', mjo.phase.values)
sp_mod_daily['n34'] = ('time', n34.classification.values)
# minimum energy to consider for counting probabilities
h_lim_cont = (0.04/4) ** 2
prob_grid = np.full(np.shape(sp_mod_daily.h), 0.0)
prob_grid[np.where(sp_mod_daily.h > h_lim_cont)] = 1
sp_mod_daily['is_h'] = (('time','t_bins','dir_bins'), prob_grid)
Forecast data El Niño3.4#
Forecast data has been downloaded from: https://www.cpc.ncep.noaa.gov/products/CFSv2/CFSv2_body.html
# download forecast MJO data
url_mjo_e1 = 'https://www.cpc.ncep.noaa.gov/products/CFSv2/dataInd1/nino34Mon.nc' # can use dataInd2 and dataInd3
with open(p_n34_forecast, 'wb') as ps:
r = requests.get(url_mjo_e1, allow_redirects=True)
ps.write(r.content)
# load downloaded file
n34_f = xr.open_dataset(p_n34_forecast)
# preprare data
n34_f = n34_f.squeeze().drop(['LON','LAT','LEVEL'])
n34_f = n34_f.sel(TIME = slice('2022-04-01','2023-01-01'))
_, n34_f = Plot_n34_forecast(n34_f, l1=0.5, l2=-0.5);
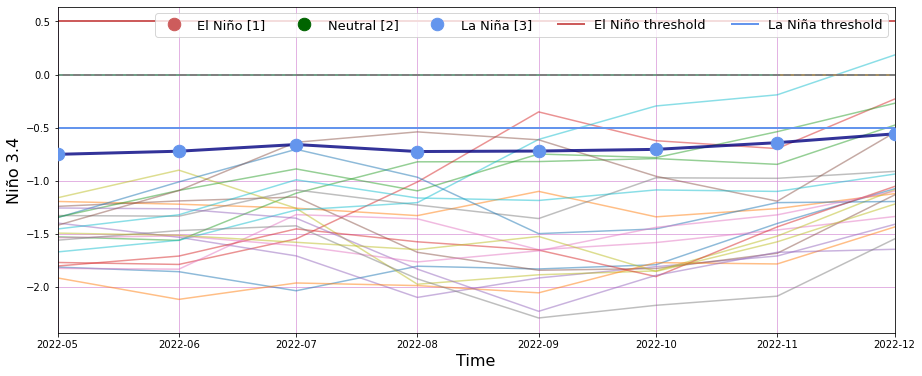
Forecast data MJO#
Forecast data can be downloaded from:
https://www.cpc.ncep.noaa.gov/products/precip/CWlink/MJO/dynverif.shtml
Forecast data for the MJO can only be obtained in a short period of time, in the order of a couple weeks, and it is not available to be introduced in a 9-month seasonal forecast
# show forecast verification
Image(url= "https://www.cpc.ncep.noaa.gov/products/precip/CWlink/MJO/operdyn_verif8D_full.gif")
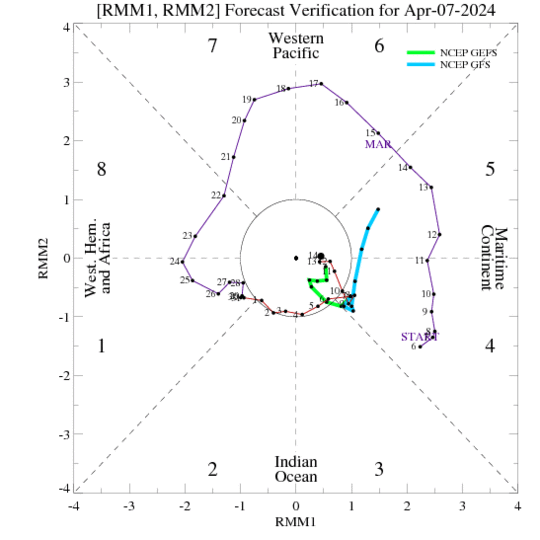
After looking at the webpage and based on the projected MJO, the forecast spectra can be obtained setting the following parameters
Daily Forecast conditioned to the MJO, the month and El Niño3.4#
Tip
Once the MJO and El niño are known, these can be manually set up here to know which are the expected conditions based on historical data.
n34_sel can be set up to 1 (El Niño), 2 (Neutral) or 3 (La Niña).
mjo_sel can be set up from 1 to 8 and corresponds to the MJO phase.
month can be set up from 1 to 12 depending on the month of the year.
After looking at the webpage and based on the projected MJO, the forecast spectra can be obtained setting the following parameters
# Parameters for the 4th May 2021
n34_sel = 3 # 1:El Niño, 2:Neutral, 3:La Niña
mjo_sel = 2 # From 1 to 8
month = 5
Conditioned to N34, MJO and Month - relative to the mean month
mean_month = 1
Plot_spec_n34_mjo_month(
sp_mod_daily,
n34_sel-1, mjo_sel, month,
an_range = 0.2,
mean_month = mean_month,
vmax_z1 = 0.9,
vmax_an = 0.04,
);
Number of data: 26
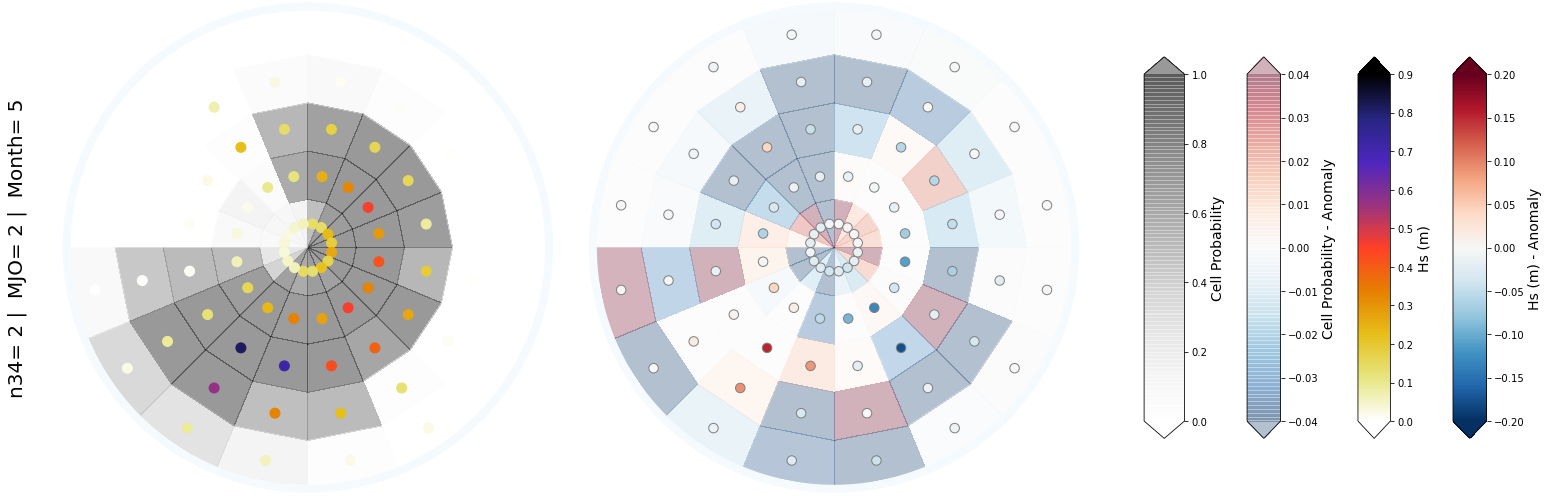
Monthly Forecast conditioned to the month and El Niño3.4#
Differently than for the MJO, forecasts of El Niño 3.4 index are available, and have been used here to develop a spectral forecast based only in the month and the category of El Niño 3.4 index.
months_list = n34_f.TIME.dt.month
n34_list = n34_f.class_ensemble.values + 1
for m in range(len(months_list)):
n34_sel = np.int(n34_list[m]-1) # 1:El Niño, 2:Neutral, 3:La Niña
mjo_sel = []
month = np.int(months_list[m])
mean_month = 1
Plot_spec_n34_mjo_month(
sp_mod_daily,
n34_sel, mjo_sel, month,
an_range = 0.2,
mean_month = mean_month,
vmax_z1 = 0.9,
vmax_an = 0.04,
)
Number of data: 308
Number of data: 404
Number of data: 365
Number of data: 448
Number of data: 561
Number of data: 451
Number of data: 372
Number of data: 241
Number of data: 250
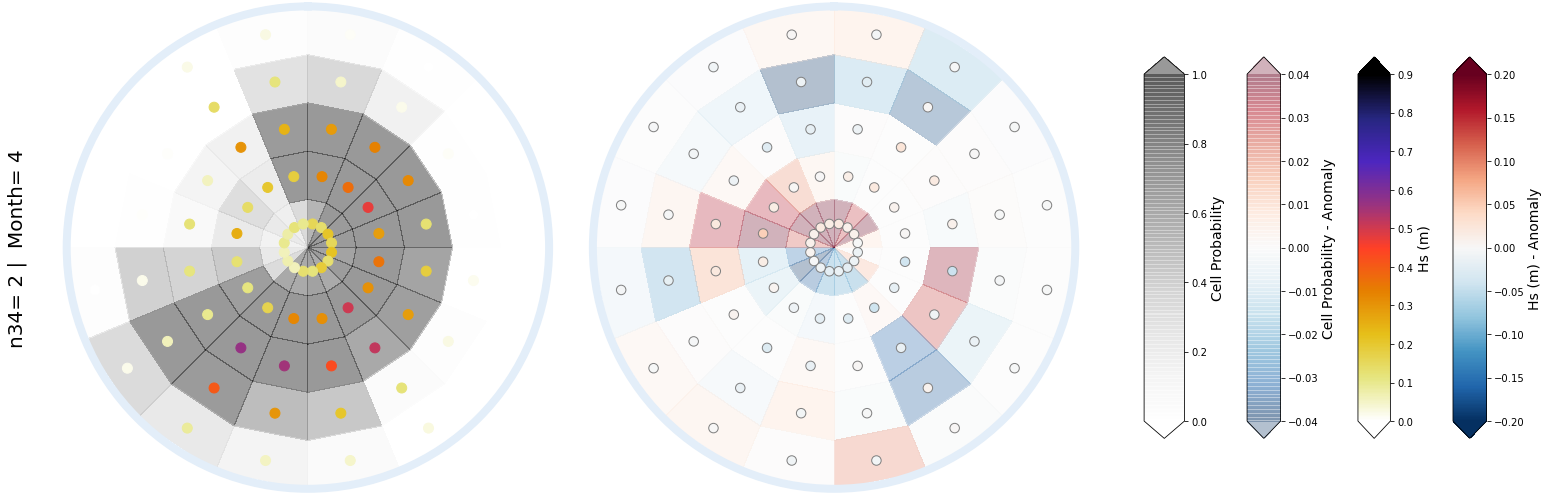
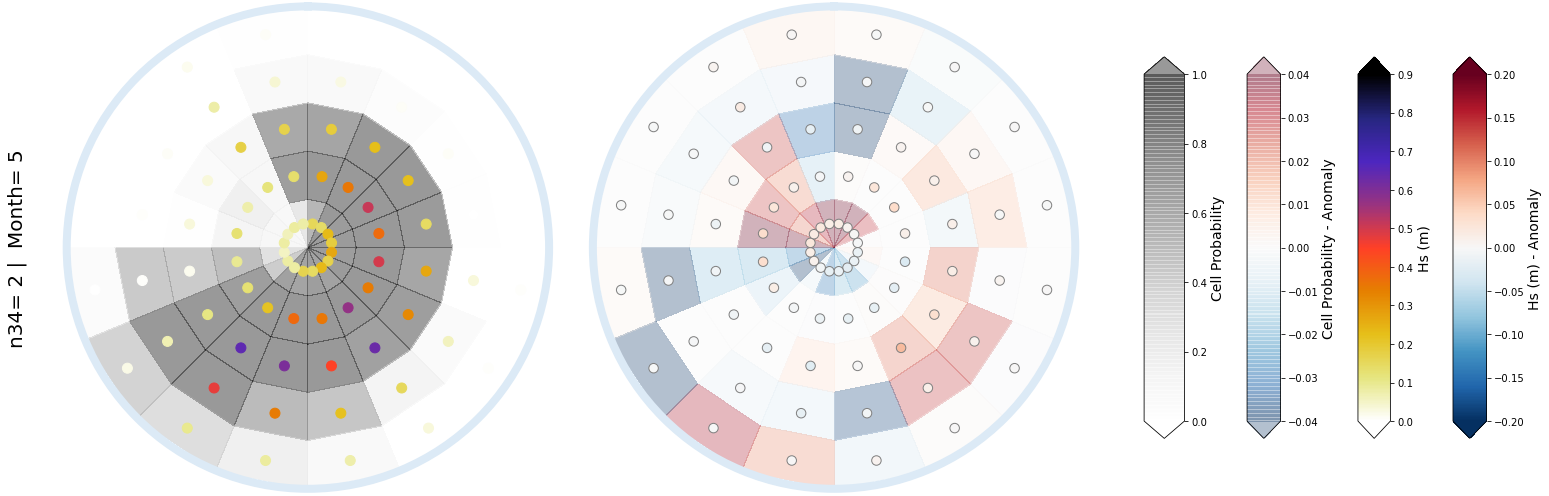
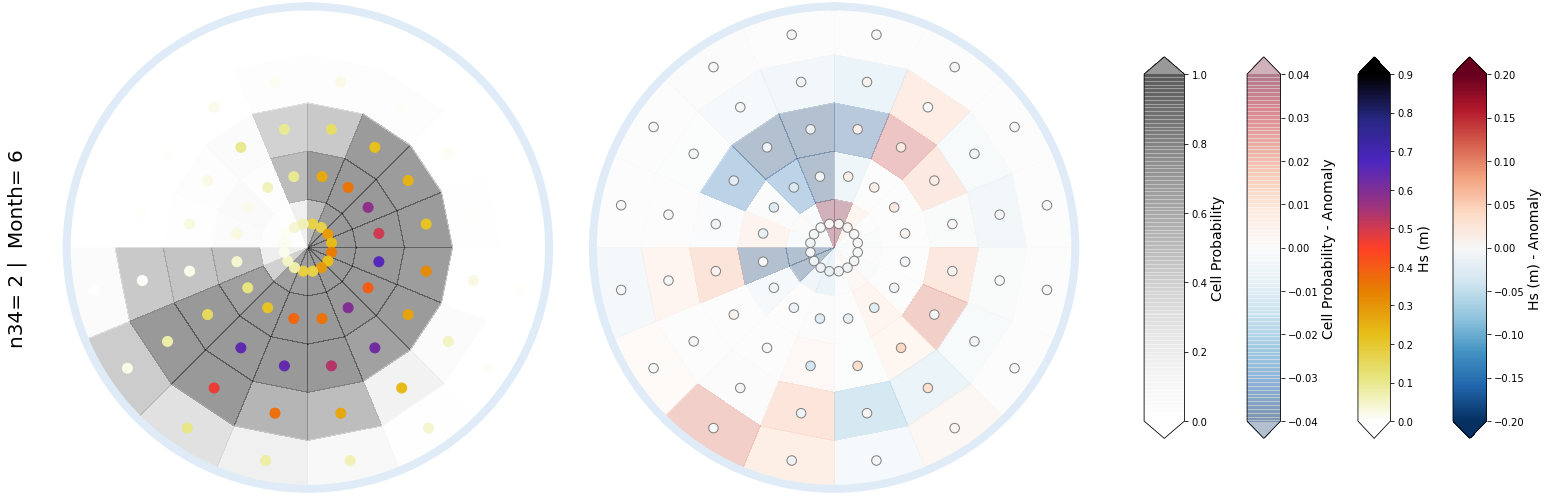
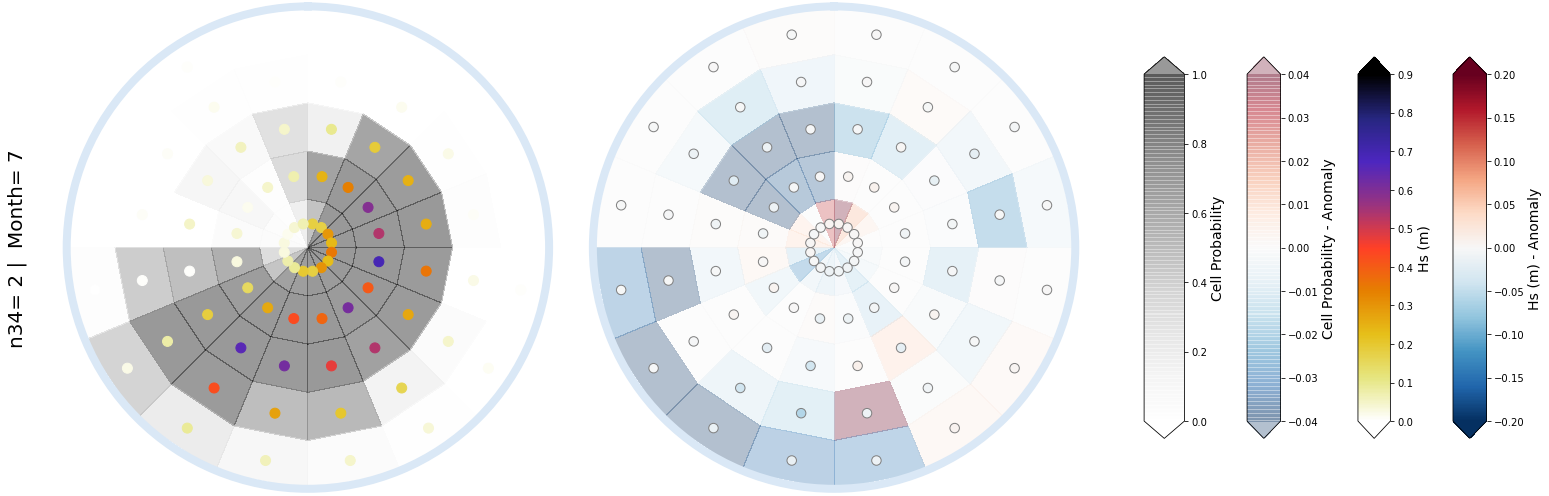
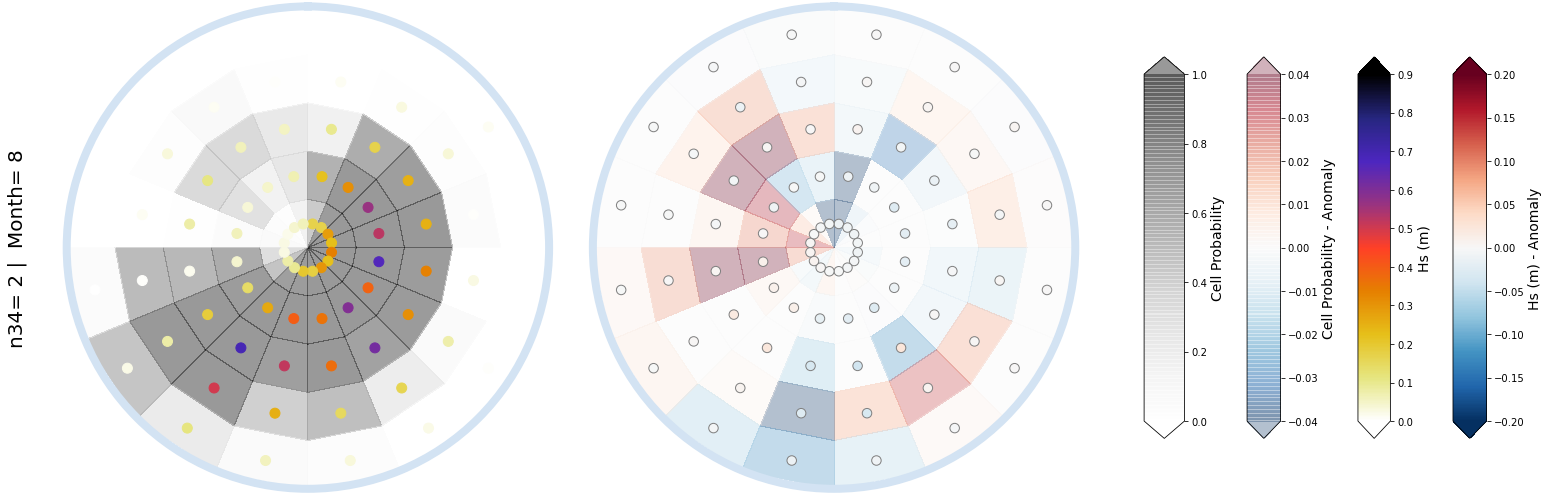
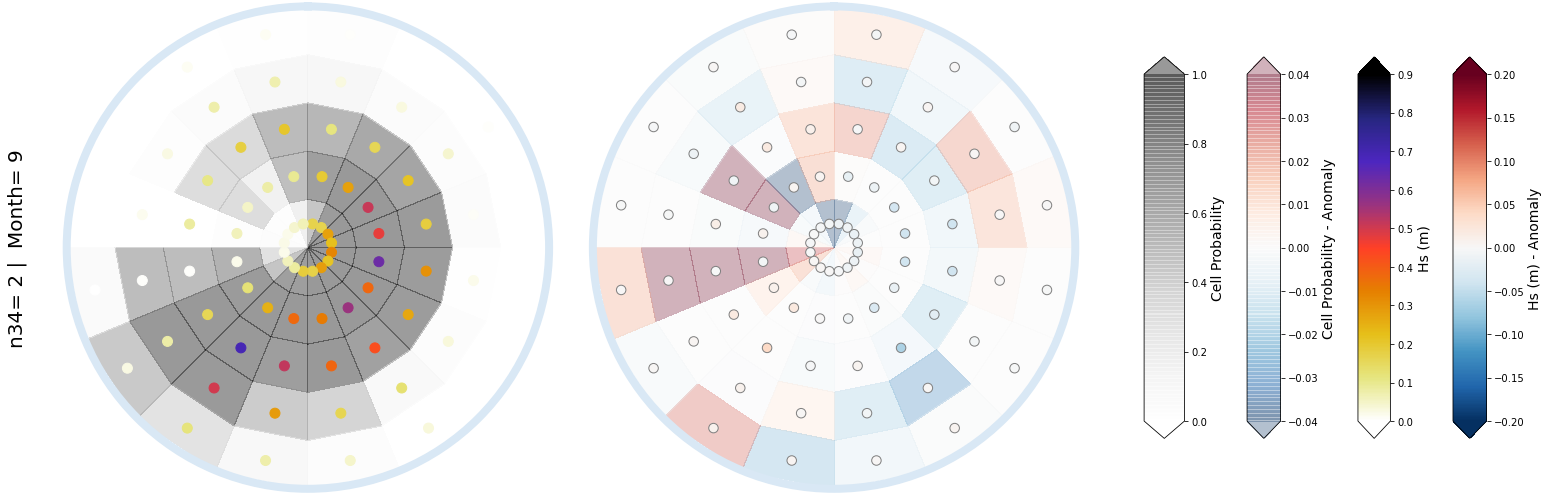
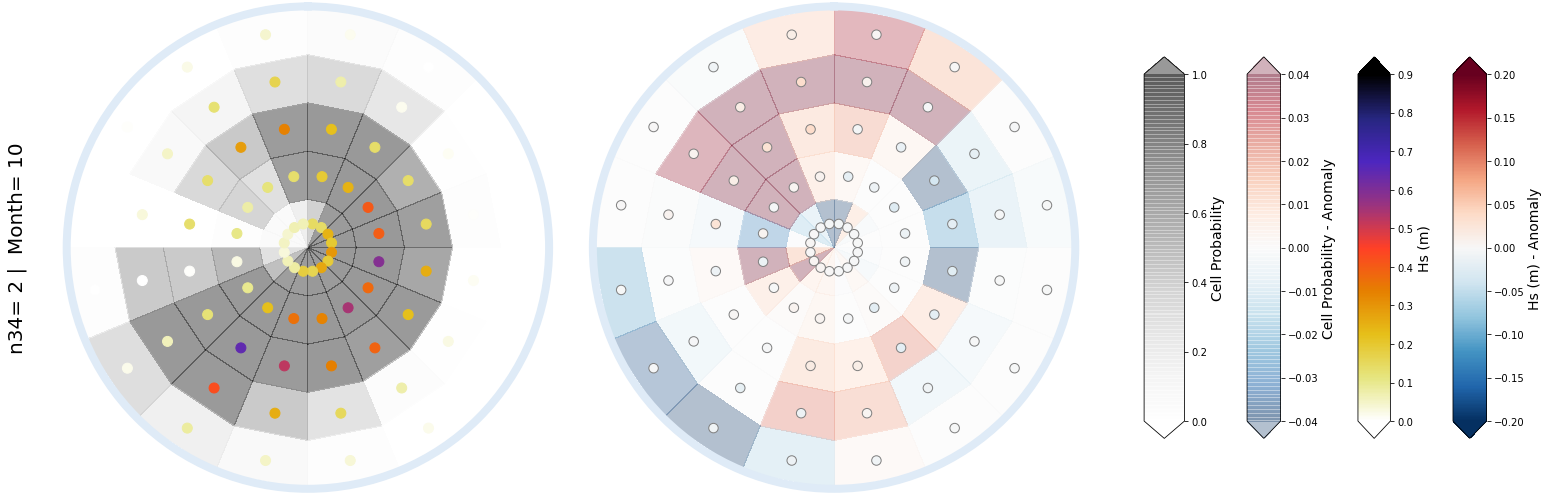
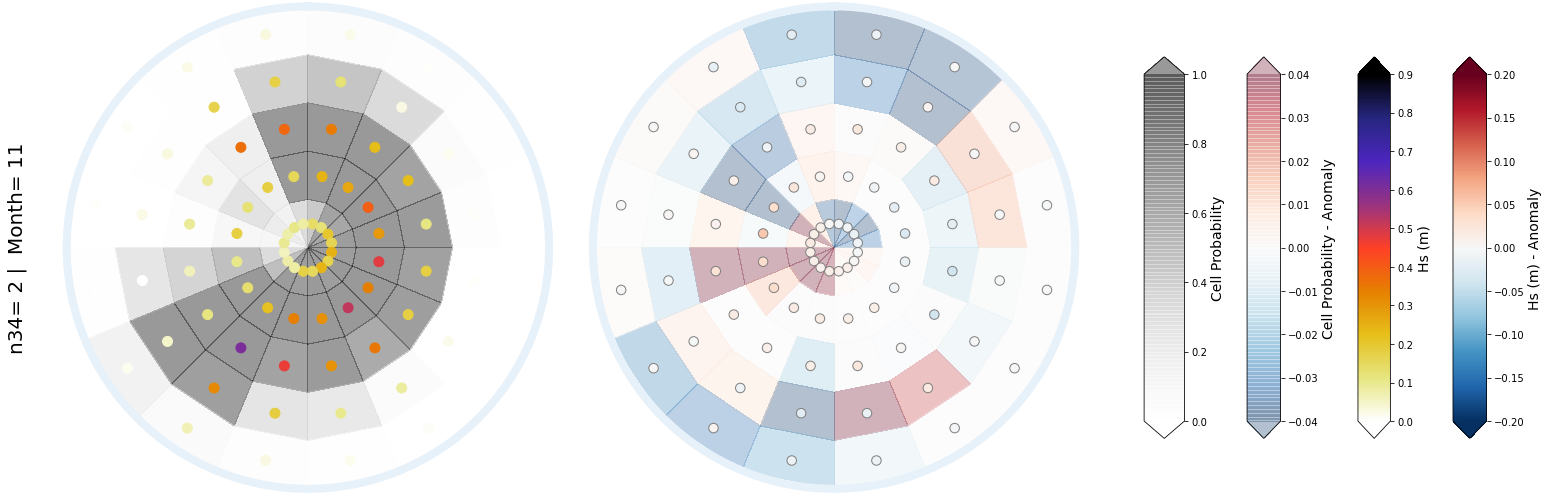
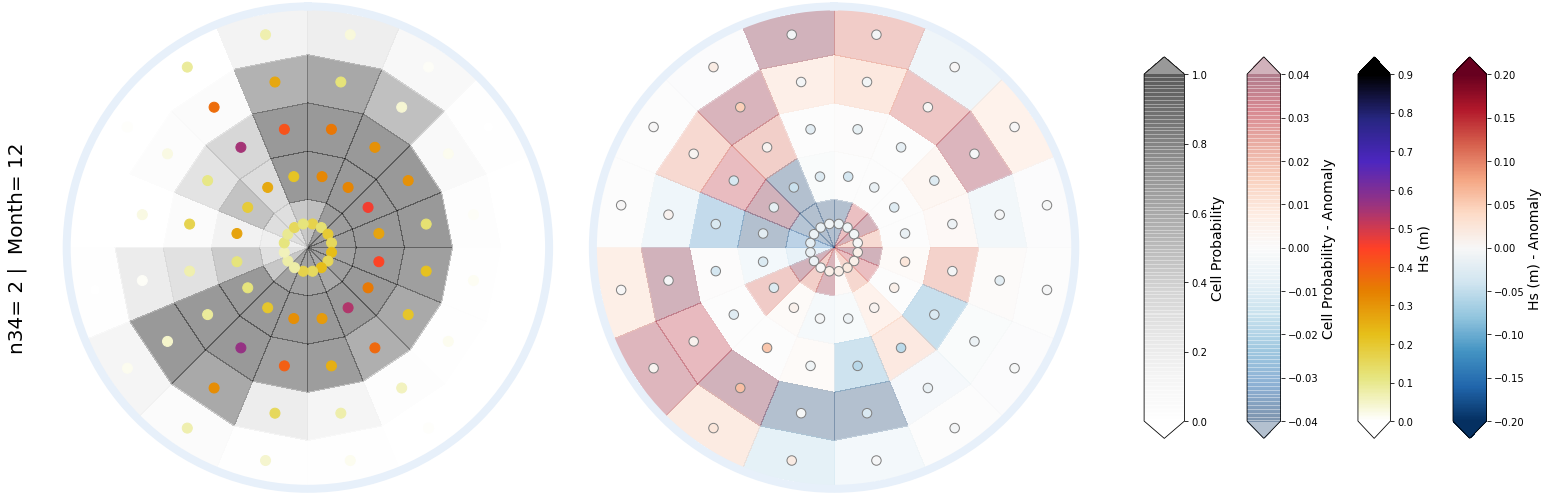