Argumentos en la línea de comando
En entra practica se verá cómo pasar argumentos en la línea de comandos a un programa Java.
Ejercicio 1: Leer argumentos desde la línea de comandos
(1.1) Construir y ejecutar
el programa Hello que recibe argumentos de la línea de comandos
1. Ir a un directorio donde se desea crear el programa.
C:\>cd
\ej_java
2. Escribir con un editor de texto (pe. notepad o jedit) el programa HelloCommandLineArguments.java como se muestra en Código-1.10.
public class HelloCommandLineArguments
{ public static void main(
String[] args ){
// Print the string "Hello,
" on screen
System.out.println("I am saying Hello to the people below..
");
// Check if a command
line argument exists
if(args.length == 0)
System.exit(0);
// Display the arguments from the command
line for(int counter = 0; counter
< args.length; counter++){
System.out.println("argument index " + counter + ": " + args[counter]);
} }
}
|
Código-1.10:
HelloCommandLineArguments.java
3. Compilar
HelloCommandLineArguments.java usando el compilador
javac.
C:\ej_java>javac HelloCommandLineArguments.java
4. Ejecutar el programa
HelloCommandLineArguments usando el
java pasando argumentos en la línea de comandos.
C:\ej_java>java HelloCommandLineArguments David Charles
Young
I am saying Hello to the people below..
argument index 0:
David
argument index 1: Charles
argument index 2: Young
Volver al inicio del ejercicio
(1.2) Leer números como argumentos de la línea de comandos
1. Ir al directorio donde se desea escribir los ficheros Java.
C:\>cd
\ej_java
2. Escribir MyCompute.java usando un editor de textos como se muestra en Código-1.20.
C:\ej_java>jedit MyCompute.java
public class MyCompute
{ public static void
main(String[] args) {
System.out.println("I am reading numbers as command line arguments..
");
// Check if a command line
argument exists
if(args.length !=
2){
System.out.println("Please enter two
numbers!");
System.exit(0);
}
// Display the addition of
the two numbers int int1 =
Integer.parseInt(args[0]);
int int2 =
Integer.parseInt(args[1]);
int additionResult = int1 +
int2;
System.out.println("Result of addition = " +
additionResult);
// Display the
multiplication of the two
numbers int1 =
Integer.parseInt(args[0]);
int2 =
Integer.parseInt(args[1]);
int multiResult = int1 *
int2;
System.out.println("Result of multiplication = " +
multiResult); }
}
|
Código-1.20: MyCompute.java
3. Compilar
MyCompute.java usando el compilador
javac.
C:\ej_java>javac MyCompute.java
4. Ejecutar el programa
MyCompute usando el comando
java pasando dos números como argumentos.
C:\ej_java>java MyCompute
I am reading numbers as
command line arguments..
Please enter two
numbers!
C:\ej_java>java
MyCompute 4
I am reading numbers as command line arguments..
Please
enter two numbers!
C:\ej_java>java MyCompute 4 6
I am reading numbers as
command line arguments..
Result of addition = 10
Result of multiplication
= 24
Volver al inicio del ejercicio
Resumen
En este ejercicio, se ha visto cómo leer argumentos en la línea de entrada de una aplicación Java.
Volver al inicio
Ejercicio 2: Leer argumentos usando NetBeans
En este ejercicio, se va a pasar argumentos a una aplicación Java utilizando NetBeans.
- Construir y ejecutar el proyecto HelloCommandLineArguments en NetBeans
- Construir y ejecutar el proyecto MyCompute en NetBeans
(2.1) Construir y ejecutar el proyecto HelloCommandLineArguments en NetBeans
1. Crear proyecto HelloCommandLineArugments.
- Seleccionar File en el menú superior y seleccionar New Project.
- Observar que aparece la ventana de diálogo de New Project.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Pulsar en Next.
- En la sección Name and Location, del campo Project Name, escribir HelloCommandLineArguments.
- En el campo Create Main Class,
escribir HelloCommandLineArguments.
- Hacer Click en Finish.
- Modificar HelloCommandLineArguments.java como se muestra en Código-2.10
public class HelloCommandLineArguments {
public static void main( String[] args ){
// Print the string "Hello, " on screen
System.out.println("I am saying Hello to the people below.. ");
// Check if a command line argument exists
if(args.length == 0)
System.exit(0);
// Display the arguments from the command line
for(int counter = 0; counter < args.length; counter++){
System.out.println("argument index " + counter + ": " + args[counter]);
}
}
} |
Código-2.10: HelloCommandLineArguments.java
2.
Paso de argumentos
- Hacer click con el botón derecho sobre el nodo del proyecto HelloCommandLineArugments
y seleccionar Properties (Figura 2.11)
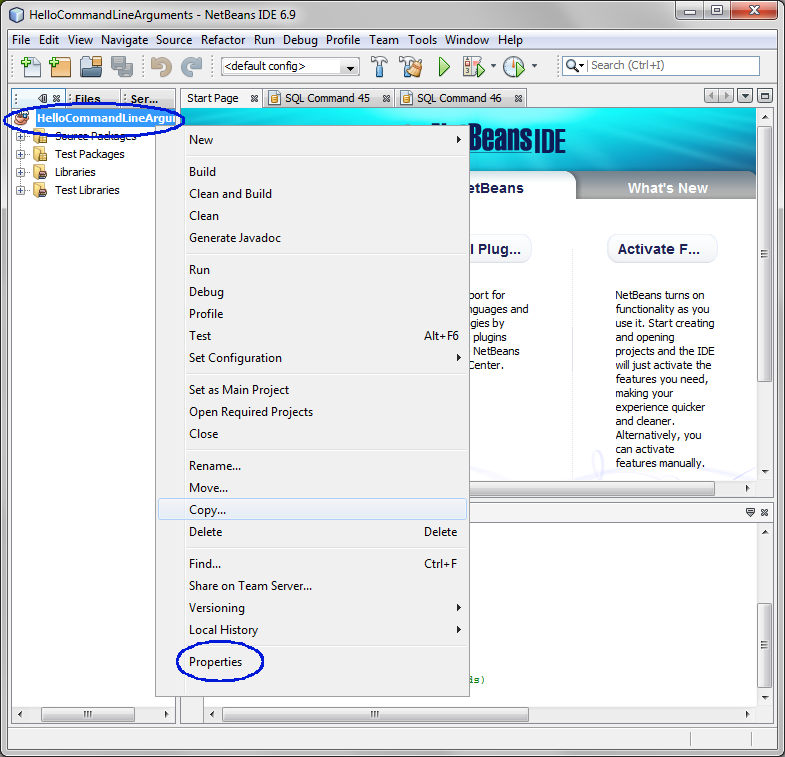
Figura-2.11: Abrir Properties del
proyecto
- Observar que aparece la ventana de diálogo Project Properties.
- Seleccionar Run en la sección Categories a la izquierda .
- Escribir en el campo Arguments en la derecha los nombres David Charles
Monica. (Figura-2.12)
- Click en OK.
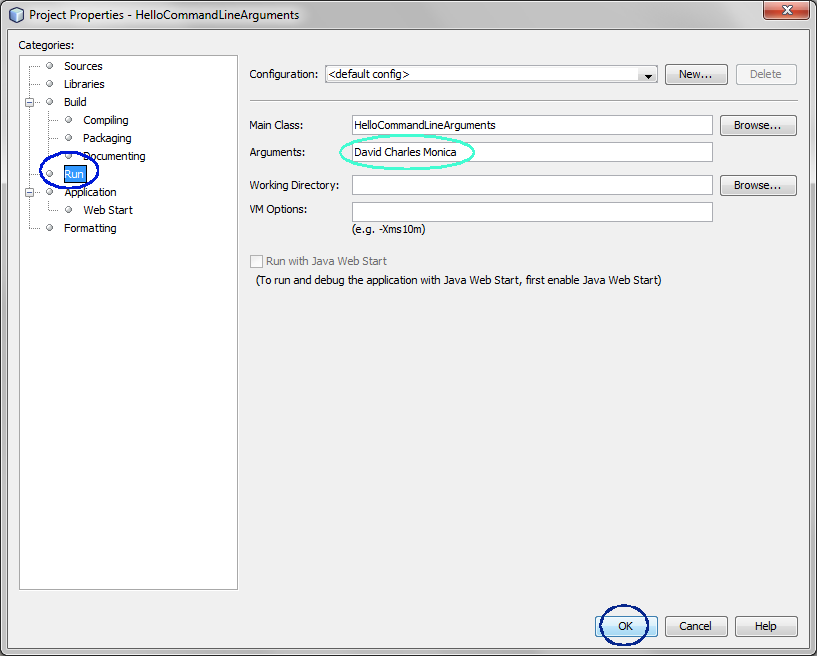
Figura-2.12: Proporcionar los argumentos de comando
3. Construir y ejecutar el proyecto.
- Hacer click con el botón derecho sobre el nodo del proyecto HelloCommandLinekArguments
y seleccionar Run.
- Observar el resultado en la ventana Output . (Figura-2.13)
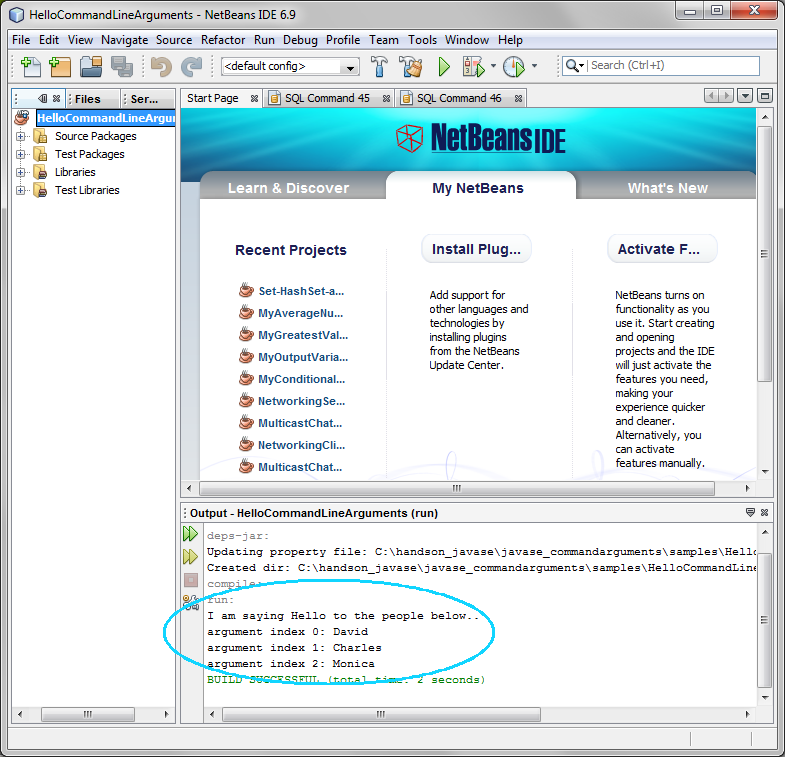
Figura-2.13: Resultado
Volver al inicio del ejercicio
(2.2) Construir y ejecutar el proyecto MyCompute
1. Crear proyecto NetBeans MyCompute.
- Seleccionar File en el menú superior y seleccionar New Project.
- Observar que aparece la ventana de diálogo de New Project.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Pulsar en Next.
- En la sección Name and Location, del campo Project Name, escribir MyCompute.
- En el campo Create Main Class,
escribir MyCompute.
- Hacer Click en Finish.
- Modificar MyCompute.java como se muestra en Código-2.21.
public class MyCompute {
public static void main(String[] args) {
System.out.println("I am reading numbers as command line arguments.. ");
// Check if a command line argument exists
if(args.length != 2){
System.out.println("Please enter two numbers!");
System.exit(0);
}
// Display the addition of the two numbers
int int1 = Integer.parseInt(args[0]);
int int2 = Integer.parseInt(args[1]);
int additionResult = int1 + int2;
System.out.println("Result of addition = " + additionResult);
// Display the multiplication of the two numbers
int1 = Integer.parseInt(args[0]);
int2 = Integer.parseInt(args[1]);
int multiResult = int1 * int2;
System.out.println("Result of addition = " + multiResult);
}
}
|
Código-2.21: MyCompute.java
2. Pasar los argumentos en la línea de comados
- Hacer click con el botón derecho sobre el nodo del proyecto MyCompute y seleccionar Properties.
- Observar que aparece el díalogo Project Properties.
- Seleccionar Run en la sección Categories.
- Escribir en el campo Arguments de la derecha los valores 10 20.
(Figura-2.21)
- Click OK.
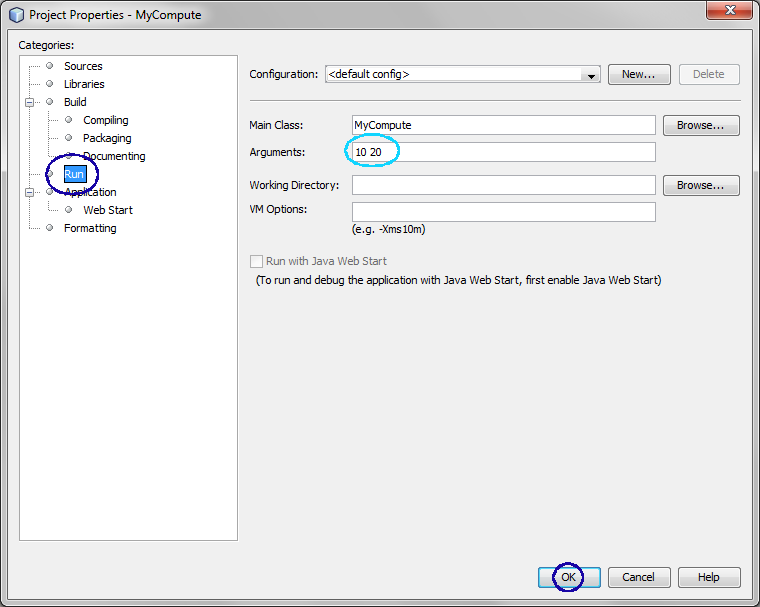
Figura-2.21: Argumentos de la línea de comandos
3. Construir y ejecutar el proyecto.
- Hacer click con el botón derecho sobre el nodo del proyecto MyCompute y seleccionar Run.
- Observar el resultado en la ventana Output del IDE. (Figura-2.13)
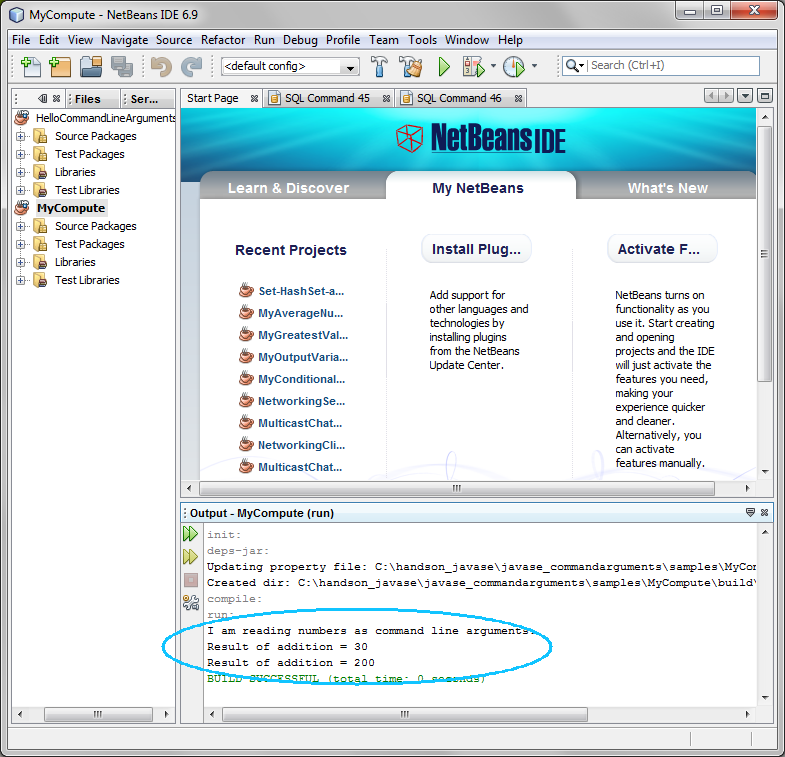
Figura-2.13:
Resultadp
Volver al inicio del ejercicio
Resumen
En este ejercicio, se ha visto cómo pasar argumentos en la línea de comando utilizando el IDE NetBeans.
Volver al inicio
Tarea
1. La tarea es crear un proyecto NetBeans llamado "MyOwnCommandLineArguments" como sigue:
- Reciba las edades de varias personas (3 a 6) como argumentos en la línea de comandos en el formato que se muestra: (nombre y edad)
- Monica 12 Daniel 34 Shelley 23
- Calcular y mostrar el promedio de las edades que fueron recibidas.