Control de flujo en Java
En esta esta práctica se va a construir y ejecutar aplicaciones que usan estructuras de control del elnguaje de programación Java tales como ciclos while o if/else.
Ejercicio 1: estructura de control if/else
En este ejercicio, se escribirá un programa Java que usa la estructura de control if/else.
(1.1) Construir y ejecutar un programa que usa la estructura de control if/else
1. Crear el proyecto NetBeans MyGradesProject.
- Seleccionar File en el menú superior y seleccionar New Project.
- Observar que aparece la ventana de diálogo de New Project.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Pulsar en Next.
- En la sección Name and Location, del campo Project Name, escribir MyGradesProject.
- En el campo Create Main Class,
escribir Grades.
- Hacer Click en Finish.
- Modificar Grades.java como se muestra en Código-1.10.
import
javax.swing.JOptionPane;
public class Grades
{ public static void
main(String[] args) {
int mathGrade =
0; int historyGrade =
0; int scienceGrade =
0; double average =
0;
mathGrade =
Integer.parseInt(JOptionPane.showInputDialog("Enter math grade between 0
and 100!")); historyGrade =
Integer.parseInt(JOptionPane.showInputDialog("Enter history grade between
0 and 100!")); scienceGrade
= Integer.parseInt(JOptionPane.showInputDialog("Enter science grade
between 0 and 100!"));
// Compute
average average =
(mathGrade+historyGrade+scienceGrade)/3;
// Perform if & else control if
(average >= 60){
JOptionPane.showMessageDialog(null, "Good job! Your average is " +
average); }
else{
JOptionPane.showMessageDialog(null, "You need to do better! Your average
is " + average);
}
} }
|
Código-1.10:
Código Grades.java
3. Construir y ejecutar el programa
- Hacer click con el botón derecho en MyGradesProject y seleccionar Run.
- Escribir notas para math, history, and science y ver el resultado. (Figura-1.12,
Figura-1.13, Figura-1.14, Figura-1.15)
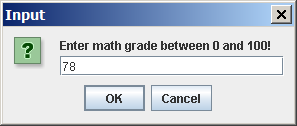
Figura-1.12: Diálogo para nota math
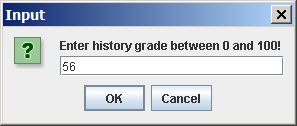
Figura-1.13: Diálogo para nota history
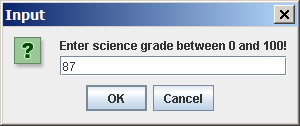
Figura-1.14: Diálogo para nota science
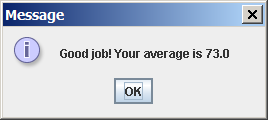
Figura-1.15: Calcula average y muestra mensajes usando if/else
4. (Ejercicio) Modificar
Grades.java
como sigue :
- Si average es mayor que 90 (average > 90), mostrar "You worked
too hard! Your average is xx.0."
- Si average es mayor que 50 (average > 50) y menor o igual a 90 (average <= 90), mostrar "You did OK! Your average is
xx.0."
- Si average es menor que o igual a 50 (average <= 50), mostrar
"You need to do some work! Your average is xx.0."
Volver al inicio del ejercicio
(1.2) Construir y ejecutar un segundo programa que usa la estructura de control if/else
1. Crear el proyecto NetBeans MyNumWordsProject.
- Seleccionar File en el menú superior y seleccionar New Project.
- Observar que aparece la ventana de diálogo de New Project.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Pulsar en Next.
- En la sección Name and Location, del campo Project Name, escribir MyNumWordsProject.
- En el campo Create Main Class,
escribir NumWords.
- Hacer Click en Finish.
- Modificar NumWords.java como se muestra en Código-1.21.
import
javax.swing.JOptionPane;
public class NumWords
{ /** Creates a new instance
of NumWords */ public NumWords()
{ }
/** * @param args the command line
arguments */ public
static void main(String[] args)
{ String msg =
""; int input =
0;
// Get the input
string input =
Integer.parseInt(JOptionPane.showInputDialog
("Enter number between 1 to
10"));
// Set msg variable to the string equivalent of
input
if(input == 1) msg = "one"; else
if(input == 2) msg = "two"; else
if(input == 3) msg = "three"; else
if(input == 4) msg = "four"; else
if(input == 5) msg = "five"; else
if(input == 6) msg = "six"; else
if(input == 7) msg = "seven"; else
if(input == 8) msg = "eight"; else
if(input == 9) msg = "nine"; else
if(input == 10) msg = "ten";
else msg =
"Invalid number";
// Display the number in
words if with in range
JOptionPane.showMessageDialog(null,msg);
} }
|
Código-1.21: NumWords.java
3. Construir y ejecutar el programa
- Hacer click con el botón derecho en MyNumWordsProject y seleccionar Run.
- Escribir un número y hacer click en OK. (Figura-1.22)
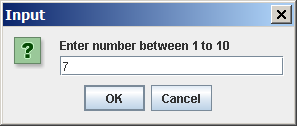
Figura-1.22: Ingresar un número
Resumen
Este ejercicio ha mostrado el uso de la estructura de control if/else.
Volver al inicio
Ejercicio 2: ciclo for
En este ejercicio, se desarrolla un programa
Java que usa el ciclo for.
- Construir y ejecutar un programa Java que usa el ciclo for
(2.1) Construir y ejecutar un programa Java que usa el ciclo for
1. Generar el proyecto MyForLoopProject.
- Seleccionar File en el menú superior y seleccionar New Project.
- Observar que aparece la ventana de diálogo de New Project.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Pulsar en Next.
- En la sección Name and Location, del campo Project Name, escribir MyForLoopProject.
- En el campo Create Main Class,
escribir ForLoop.
- Hacer Click en Finish.
- Modificar ForLoop.java como se muestra en Código-2.21.
import
javax.swing.JOptionPane;
public class ForLoop
{ /** Creates a new instance
of ForLoop */ public ForLoop()
{ }
/** * @param args the command line
arguments */ public
static void main(String[] args)
{ // Declare and initialize
String array variable called
names. String names
[]={"Beah","Bianca","Lance","Belle","Nico","Yza","Gem","Ethan"};
// This is the search
string we are going to use to search the
array. String searchName =
JOptionPane.showInputDialog("Enter either \"Yza\" or
\"noname\"!");
// Declare and initialize
boolean primitive type variable called
foundName. boolean foundName
=false;
// Search the
String array using for loop.
// * The "names.length" is the size of the array.
// * This for loop compares the value of each entry of the array
with
// the value of searchString String type
variable.
// * The equals(..) is a method of String class. Think about why
you
// cannot use "names[i] == searchName" as
comparison logic here.
for
(int i=0; i<names.length; i++){
if (names [i ].equals(searchName)){
foundName =true;
break;
}
}
// Display the
result if
(foundName)
JOptionPane.showMessageDialog(null, searchName + " is
found!");
else
JOptionPane.showMessageDialog(null, searchName + " is not
found!");
}
}
|
Código-2.21: ForLoop.java
3. Construir y ejecutar el programa
- Hacer click con el botón derecho en MyForLoopProject y seleccionar Run.
- Ingresar Yza que es una de las cadenas contenidas en el array. (Figura-2.22)
- Observar que Yza es localizada dentro del ciclo for de búsqueda. (Figura-2.23)
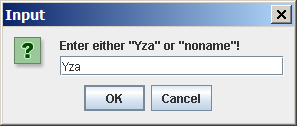
Figura-2.22: Ingreasr cadena a buscar
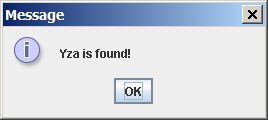
Figura-2.23: Yza encontrada
- Hacer click con el botón derecho en MyForLoopProject y seleccionar Run.
- Ingresar noname que no es una cadena almacenada en el array. (Figura-2.24)
- Observar que noname no se encuentra dentro del ciclo for de búsqueda. (Figura-2.25)
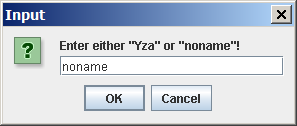
Figura-2.24: Ingresar cadena a buscar
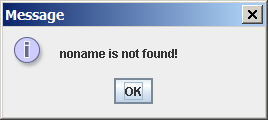
Figura-2.25: noname no se encuentra
Resumen
Este ejercicio ha mostrado el uso del ciclo for.
Volver al inicio
Ejercicio 3: ciclo while
En este ejercicio se desarrolla un programa que usa el ciclo while y do-while.
- Construir y ejecutar un programa que usa ciclo while
- Construir y ejecutar un programa que usa ciclo do-while
(3.1) Construir y ejecutar un programa que usa ciclo while
1. Crear proyecto MyFiveNamesUsingwhileProject.
- Seleccionar File en el menú superior y seleccionar New Project.
- Observar que aparece la ventana de diálogo de New Project.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Pulsar en Next.
- En la sección Name and Location, del campo Project Name, escribir MyFiveNamesUsingwhileProject.
- En el campo Create Main Class,
escribir FiveNamesUsingwhile.
- Hacer Click en Finish.
- Modificar FiveNamesUsingwhile.java como se muestra en Código-3.11.
import java.io.BufferedReader; import
java.io.InputStreamReader;
public class FiveNamesUsingwhile
{
/** * Creates a new instance of
FiveNamesUsingwhile */
public FiveNamesUsingwhile() {
}
/** * @param args the command line
arguments */ public
static void main(String[] args)
{ BufferedReader reader
= new BufferedReader(new
InputStreamReader(System.in));
String name = ""; int
counter = 0;
//gets the users'
name
try{
System.out.println("Enter name:
");
name = reader.readLine();
}catch(Exception
e){
System.out.println("Invalid
input");
System.exit(0);
} //
while loop that prints the name five times while
(counter < 5){
System.out.println(name);
counter++;
} } }
|
Código-3.11:
FiveNamesUsingwhile.java
3. Construir y ejecutar el programa
- Hacer click con el botón derecho en MyFiveNamesUsingwhileProject y seleccionar Run.
- El programa solicita introcucir un nombre Enter
name: y pulsar la tecla Enter.
(Figura-3.12)
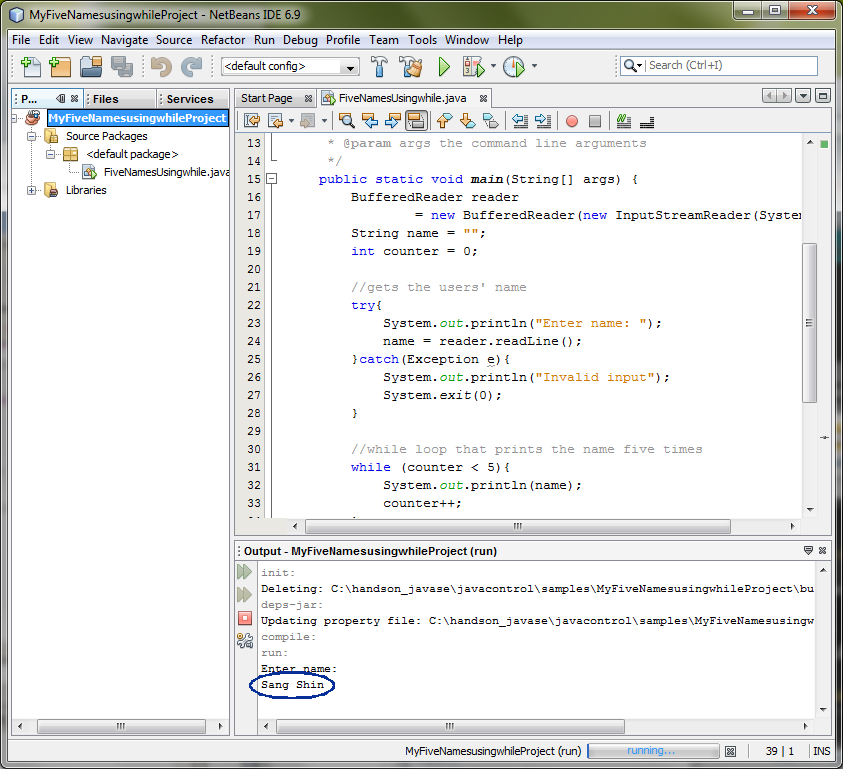
Figura-3.12: Ingresar un nombre
- Observar que el nombre se repite 5 veces (Figura-3.13 ).
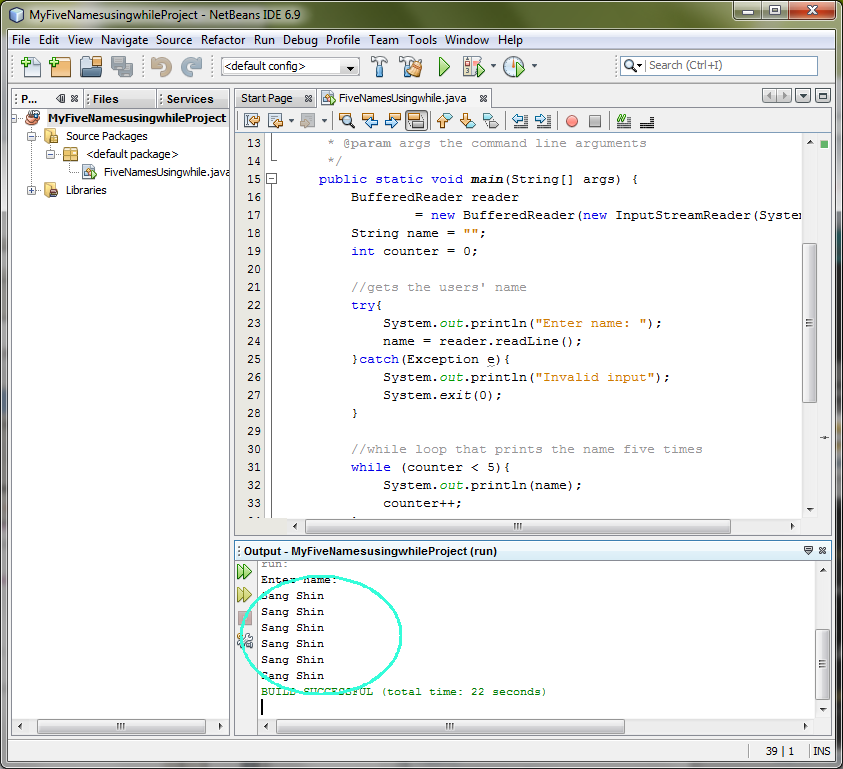
Figura-3.13: Name se repite 5 veces usando el ciclo while
Volver al inicio del ejercicio
(3.2) Construir y ejecutar un programa que usa ciclo do-while
1. Crear el proyecto MyFiveNamesUsingdowhileProject en NetBeans.
- Seleccionar File en el menú superior y seleccionar New Project.
- Observar que aparece la ventana de diálogo de New Project.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Pulsar en Next.
- En la sección Name and Location, del campo Project Name, escribir MyFiveNamesUsingdowhileProject
- En el campo Create Main Class,
escribir FiveNamesUsingdowhile.
- Hacer Click en Finish.
- Modificar FiveNamesUsingdowhile.java como se muestra en Código-3.21.
import java.io.BufferedReader; import
java.io.InputStreamReader;
public class FiveNamesUsingdowhile
{ public static void
main(String[] args){
BufferedReader
reader
= new BufferedReader(new
InputStreamReader(System.in));
String name = ""; int
counter = 0;
//gets the users'
name
try{
System.out.println("Enter name:
");
name = reader.readLine();
}catch(Exception
e){
System.out.println("Invalid
input");
System.exit(0);
}
// Use do-while loop that prints the name five
times
do{
System.out.println(name);
counter++;
}while(counter < 5);
} }
|
Código-3.21:
FiveNamesUsingdowhile.java
3. Construir y ejecutar el programa
- Hacer click con el botón derecho en MyFiveNamesUsingdowhileProject y seleccionar Run.
- Observar que el programa pide ingresar name.
- Escribir un nombre en el campo Input y pulsar la tecla Enter.
- Name debe repetirse 6 veces.
Volver al inicio del ejercicio
Resumen
En este ejercicio se ha usado los ciclos while y
do-while loop.
Tarea
1. La tarea consiste en modificar el proyecto MyForLoopProject (Ejercicio 2) utilizando en lugar del ciclo for un ciclo while (se sugiere crear otro proyecto, ejemplo MyOwnWhileProject).