Elementos de Java
El objetivo de este laboratorio es presentar los elementos básicos del lenguaje de programación Java, tales como la declaración y uso de variables y operadores.
Ejercicios de Laboratorio
Ejercicio 1: Declarar, inicializar, imprimir variables
En este Ejercicio, se va a declarar e inicializar un a variable. También se muestra cómo modificar y mostrar el valor de una variable.
(1.1) Construir (Build) y ejecutar (Run) el programa Java OutputVariable utilizando NetBeans IDE
0. Iniciar NetBeans IDE si no lo está.
1. Crear el proyecto
- Seleccionar File en el menú superior y seleccionar New Project.
- Observar que aparece la ventana de diálogo de New Project.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Pulsar en Next.
- En la sección Name and Location, del campo Project Name, escribir MyOutputVariableProject.
Este es el nombre que se le da al nuevo proyecto creado con NetBeans.
- En el campo Create Main Class,
escribir OutputVariable.
(Figura-1.10) Esto es para crear OutVariable.java, en el que el método main(..) será creado de forma automática.
- Hacer Click en Finish.
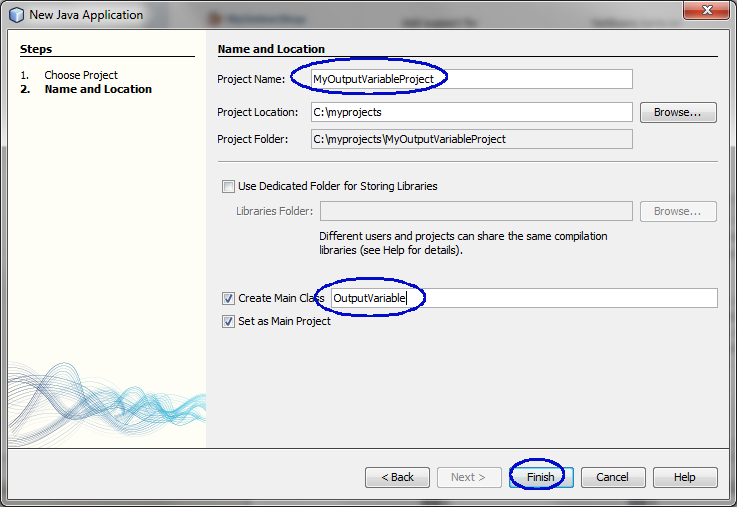
Figura-1.10: Creación del proyecto OutVariable
- Observe que el nodo del proyecto MyOutputVariableProject
se crea bajo el panel Projects
del IDE NetBeans y que el fichero OutputVariable.java se muestra en la ventana del editor del IDE.
2. Modificar el fichero generado
OutputVariable.java.
- Modificar el OutputVariable.java como se muestra en Código-1.11 y la Figura-1.12 de abajo. Los fragmentos de código que necesitan ser agregados esán resaltados en negrita y color azul.
/* *
OutputVariable.java *
* Created on January 19, 2010,
6:30 PM
* * To change this template, choose Tools |
Template Manager * and open the template in the
editor. */
/** * * @author
*/ public class OutputVariable {
/** * @param args the
command line arguments
*/ public static void main(String[] args)
{
// Variable value is int primitive type and it is initialized to 10
int value = 10;
// Variable x is char primitive type and it is initialized to 'A'
char x;
x = 'A';
// Variable grade is a double type
double grade = 11;
// Display the value of variable "value" on the standard output device
System.out.println( value );
//Display the value of variable "x" on the standard output device
System.out.println( "The value of x=" + x );
// Display the value of variable "grade" on the standard output device
System.out.println( "The value of grade =" + grade
); }
}
|
Código-1.11: Modificación de
OutputVariable.java
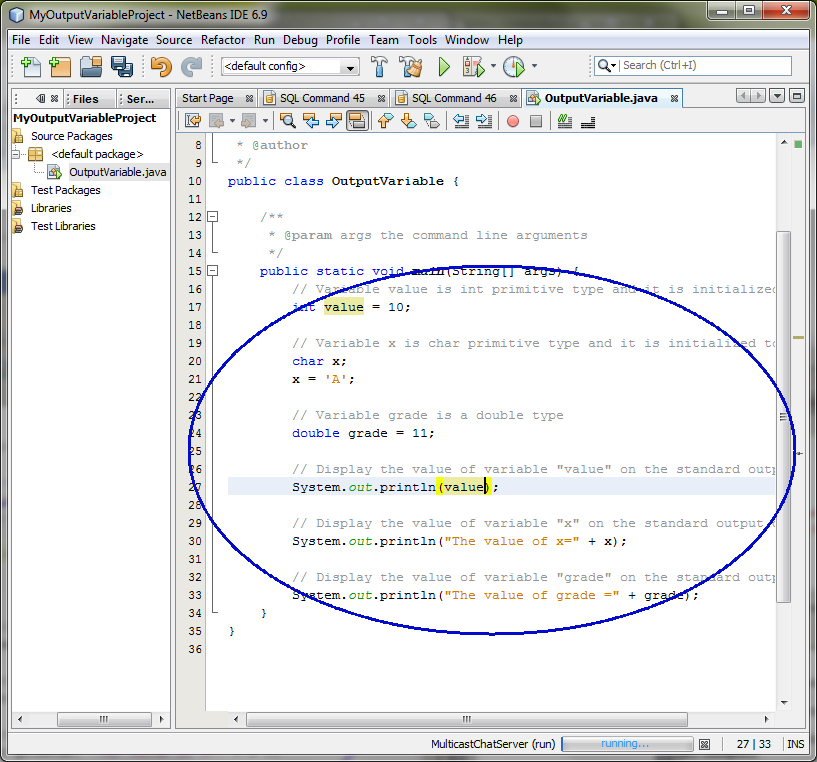
Figura-1.12: Modificación de
OutputVariable.java
3. Construir (Build) y ejecutar (run) el programa
- Seleccionar con el botón derecho MyOutputVariableProject
y seleccionar Run.
- Observar el resultado en la ventana Output
del IDE NetBeans. (Figura-1.13)
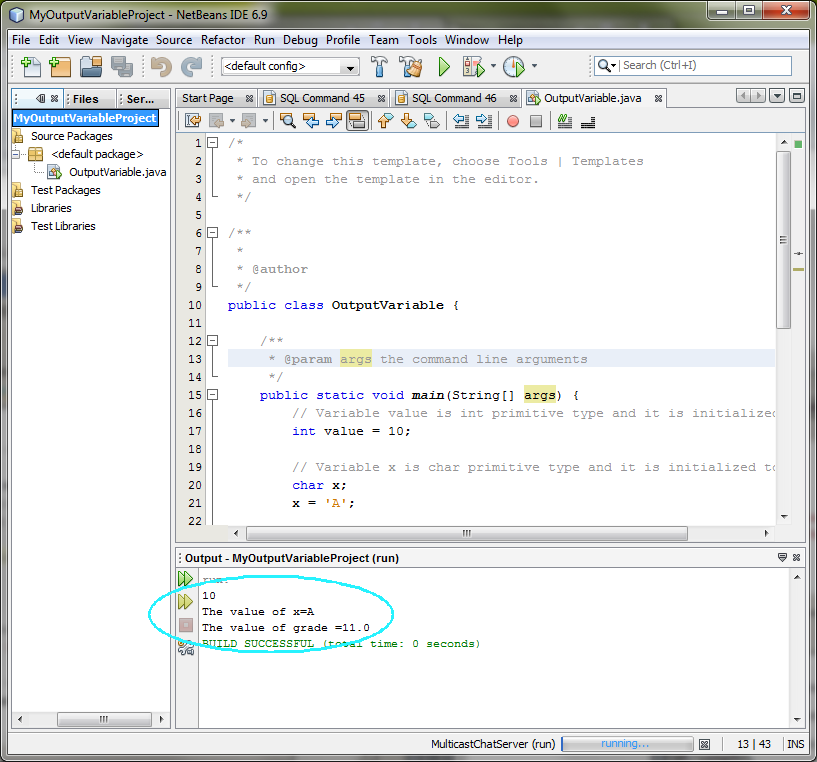
Figura-1.13: Resultado de ejecutar el programa
OutputVariable
Volver al inicio del Ejercicio
(1.2) Construir (Build) y ejecutar (Run) el programa
OutputVariable usando el compilador "javac" y "java"
1. Ir al directorio donde se va a escribir los programas Java
C:\>cd
\myjavaprograms
2. Escribir OutputVariable.java usando el editor de su preferencia
(en este ejemplo se usa jedit) como se muestra en Código-1.20.
C:\myjavaprograms>jedit OutputVariable.java
public class OutputVariable
{
public static void main( String[] args
){
// Variable value is
int primitive type and it is initialized to
10 int value =
10;
// Variable x is char
primitive type and it is initialized to
'A' char
x; x =
'A';
// Display the value of
variable "value" on the standard output
device System.out.println(
value );
// Display the
value of variable "x" on the standard output
device System.out.println(
"The value of x=" + x );
}
} |
Código-1.20: OutputVariable.java
3.
Compilar
OutputVariable.java usando el compilador
javac. El resultado de la compilación es la creación del fichero
OutputVariable.class.
C:\myjavaprograms>javac OutputVariable.java
4. Ejecutar el programa
OutputVariable usando el comando
java.El comando
java inicia la Java Virtual Machine
y ejecuta el programa
OutputVariable en este ejemplo. Un programa Java puede estar compuesto de múltiples clases Java y un conjunto de librerías. En este ejemplo, el programa OutputVariable sólo contiene una clase simple llamada
OutputVariable.class. Se puede refereir al comando
java como un intérprete de Java.
C:\myjavaprograms>java OutputVariable
10
The value of
x=A
7. Modificar
OutputVariable.java como se muestra en Código-1.21
abajo. La modificación es para añadir la variable de tipo double llamada grade y mostrar el valor de la misma. Los fragmentos de código que necesitan ser agregados esán resaltados en negrita y color azul.
public class OutputVariable
{
public static void main( String[] args
){
// Variable value is
int primitive type and it is initialized to
10 int value =
10;
// Variable x is char
primitive type and it is initialized to
'A' char
x; x =
'A';
// Variable grade is a double type
double grade = 11;
// Display the value of
variable "value" on the standard output
device System.out.println(
value );
// Display the
value of variable "x" on the standard output
device System.out.println(
"The value of x=" + x );
//
Display the value of variable "grade" on the standard output
device
System.out.println( "The value of grade =" + grade
);
}
}
|
Código-1.21: Modificación de OutputVariable.java
8.
Compilar y ejecutar el programa. Observar el nuevo mensaje mostrado.
C:\myjavaprograms>javac
OutputVariable.java
C:\myjavaprograms>java OutputVariable
10
The value of
x=A
The value of grade =11.0
Resumen
En este ejercicio, se ha construído y ejecutado el programa Java OutputVariable usando el compilador javac y el comando java y el IDE NetBeans.
Volver al inicio
Ejercicio 2: Operador condicional
En este ejercicio, se va a escribir un programa Java
que usa operadores condicionales.
- Construir y ejecutar un programa Java que usa operadores condicionales
(2.1) Compilar y ejecutar un programa Java que usa operadores condicionales
1. Crear un proyecto NetBeans
- Seleccionar File del menú principal y seleccionar New Project.
- Observar que el diálogo New Project aparece.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Click en Next.
- En el panel Name and Location, en el campo Project Name, escribir MyConditionalOperatorProject.
- En el campo Create Main Class,
ingresar ConditionalOperator.
- Click en Finish.
- Observar que el nodo MyConditionalOperatorProject del proyecto es creado en el panel Projects del IDE
NetBeans y que aparece ConditionalOperator.java en la ventana del editor del IDE.
2. Modificar el fichero ConditionalOperator.java generado.
- Modificar ConditionalOperator.java
como se muestra abajo en Código-2.21. Los fragmentos de código que necesitan ser agregados esán resaltados en negrita y color azul.
public class ConditionalOperator
{
/** * @param args the command line
arguments */ public
static void main(String[] args) {
// Declare and initialize two variables, one
String type variable
// called status and the other int primitive type variable called
// grade.
String status = "";
int grade = 80;
// Get status of the student.
status = (grade >= 60)?"Passed":"Fail";
// Print status.
System.out.println( status );
} }
|
Código-2.21: Modificación de
ConditionalOperator.java
3. Construir (Build) y ejecutar (run) el programa
- Seleccionar con el botón derecho MyConditionalOperatorProject
y seleccionar Run.
- Observar el resultado en la ventana Output
del IDE NetBeans. (Figura-2.22)
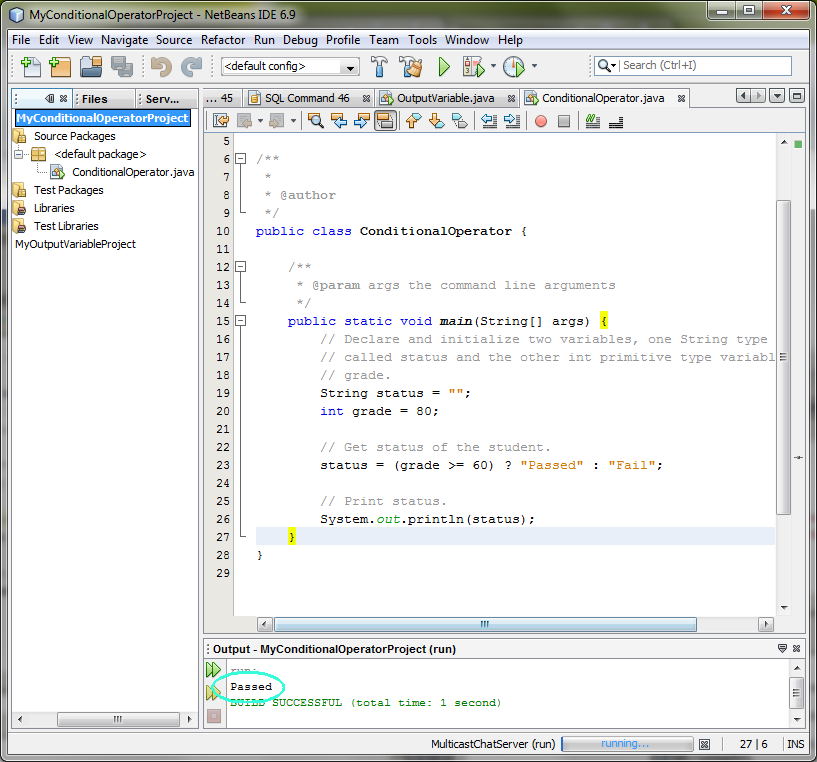
Figura-2.22: Resultado de ejecución del programa
4. Modificar ConditionalOperator.java añadiendo las siguientes líneas de código en el lugar apropiado, construir (Build) y ejecutar (run) el programa
- int salary = 100000;
- Imprimir "You are rich!" si salary es mayor a 50000. Imprimir "You are poor!"
en otro caso.
Resumen
En este ejercicio, se ha desarrollado una aplicación Java usando el IDE NetBeans.
Volver al inicio
Ejercicio 3: Promedio de números
En este ejercicio, you are going to build and run a
sample Java program in which an average of numbers are computed and displayed.
- Construir y ejecutar un programa Java
(3.1) Construir y ejecutar un programa Java
1. Crear un proyecto NetBeans
- Seleccionar File en el menú superior y seleccionar New Project.
- Observar que el diálogo New Project aparece.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Click Next.
- En el panel Name and Location, en el campo Project Name, escribir MyAverageNumberProject.
- En el campo Create Main Class,
escribir AverageNumber.
- Click Finish.
- Observar que se crea el nodo MyAverageNumberProject
en el panel Projects
del IDE NetBeans y que el IDE genera AverageNumber.java en la ventana del editor.
2. Modificar el código generado AverageNumber.java.
- Modificar AverageNumber.java como se muestra en Código-3.11 abajo. Los fragmentos de código que necesitan ser agregados esán resaltados en negrita y color azul.
public class AverageNumber
{
/** * @param args the command line
arguments */ public
static void main(String[] args) {
//declares the three numbers
int num1 = 10;
int num2 = 20;
int num3 = 45;
//get the average of the three numbers
// and saves it inside the ave variable
int ave = (num1+num2+num3)/3;
//prints the output on the screen
System.out.println("number 1 = "+num1);
System.out.println("number 2 = "+num2);
System.out.println("number 3 = "+num3);
System.out.println("Average is = "+ave);
} }
|
Código-3.11: Modificación de
AverageNumber.java
3. Construir (Build) y ejecutar (run) el programa
- Seleccionar con el botón derecho MyAverageNumberProject
y seleccionar Run.
- Observar el resultado en la ventana Output del IDE NetBeans. (Figura-3.12)
Ejercicio 4: Hallar el mayor número
En este ejercicio, se escribirá un programa Java que usa operadores condicionales.
- Construir y ejecutar un programa Java que usa operadores condicionales
(4.1) Construir y ejecutar un programa Java que usa operadores condicionales
1. Crear un proyecto NetBeans
- Seleccionar File en el menú principal y seleccionar New Project.
- Observar que aparece el diálogo New Project.
- Seleccionar Java en la sección Categories y Java Application en la sección Projects.
- Click Next.
- En el panel Name and Location, para el campo Project Name escribir MyGreatestValueProject.
- En el campo Create Main Class,
escribir GreatestValue.
(Figura-4.10)
- Click Finish.
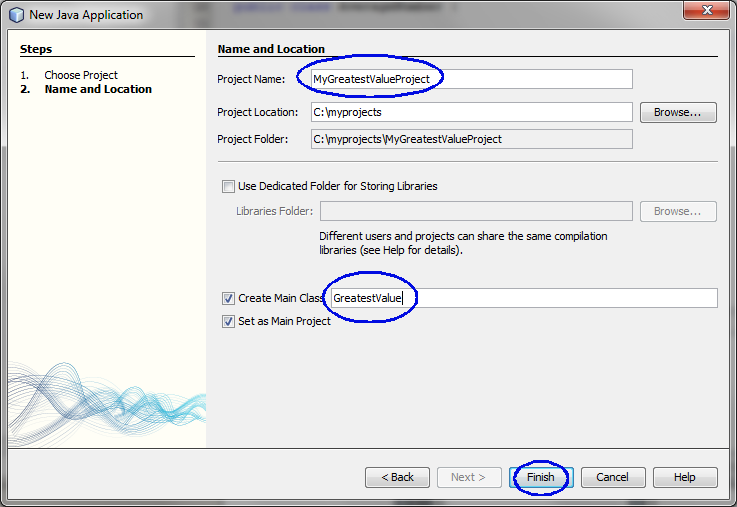
Figura-4.10: Creación de MyGreatestValueProject
- Observar que el nodo del proyecto MyGreatestValueProject
se crea en la sección Projects
del IDE de NetBeans y que se genera y muestra GreatestValue.java en la ventana del editor.
2. Modifcar el código generado GreatestValue.java.
- Modificar GreatestValue.java como se muestra en Código-4.11. Los fragmentos de código que necesitan ser agregados esán resaltados en negrita y color azul.
public class GreatestValue
{
/** * @param args the command line
arguments */ public
static void main(String[] args) {
//declares the numbers
int num1 = 10;
int num2 = 23;
int num3 = 5;
int max = 0;
//determines the highest number
max = (num1>num2)?num1:num2;
max = (max>num3)?max:num3;
//prints the output on the screen
System.out.println("number 1 = "+num1);
System.out.println("number 2 = "+num2);
System.out.println("number 3 = "+num3);
System.out.println("The highest number is =
"+max); }
}
|
Código-4.11: GreatestValue.java modificado
3. Construir (Build) y ejecutar (run) el programa
- Seleccionar con el botón derecho MyGreatestValueProject
y seleccionar Run.
- Observar el resultado en la ventana Output del IDE NetBeans. (Figura-4.12)
Tarea
1. La tarea es modificar el proyecto MyGreatestValueProject anterior. Se puede nombrar de cualquier manera al proyecto, pero aquí se le llama MyGreatestValueProject2.
- Calcular el menor número y mostrarlo
- Si el menor número es menor que 10, mostrar el mensaje: "El menor número es menor que 10!". En otro caso, mostrar "El menor número es mayor o igual a 10!".